In a prior blog post, we described how to set up an Integration Builder FaaR (Flow as a Resource). This allows us to use the 170+ connectors and flow editor to create graphically APIs.
In this blog post, we’ll create a more complex aggregate API. The API will expect a query parameter for a Salesforce Account ID and return the Account details and related Contacts.
We will create a data response that will be in the form of:
{ "account": { "Name": "Burlington Textiles Corp of America", "NumberOfEmployees": 9000, "Industry": "Apparel", . . . }, "contacts": [ { "Name": "John Doe", "MobilePhone": "+16176428274", . . . }, { "Name": "Jim Doe", "MobilePhone": "+16175551212", . . . } ] }
Let’s get started.
Create your FaaR
- Since we’re using Salesforce as our data source you will need to create a connector to your Salesforce instance. This has been covered in several prior Integration Builder blog posts.
Follow the instructions in the prior blog post, to create a new FaaR. I called mine sfdc aggregate faar and it has an API URI of /accountwithcontacts and is a GET API:
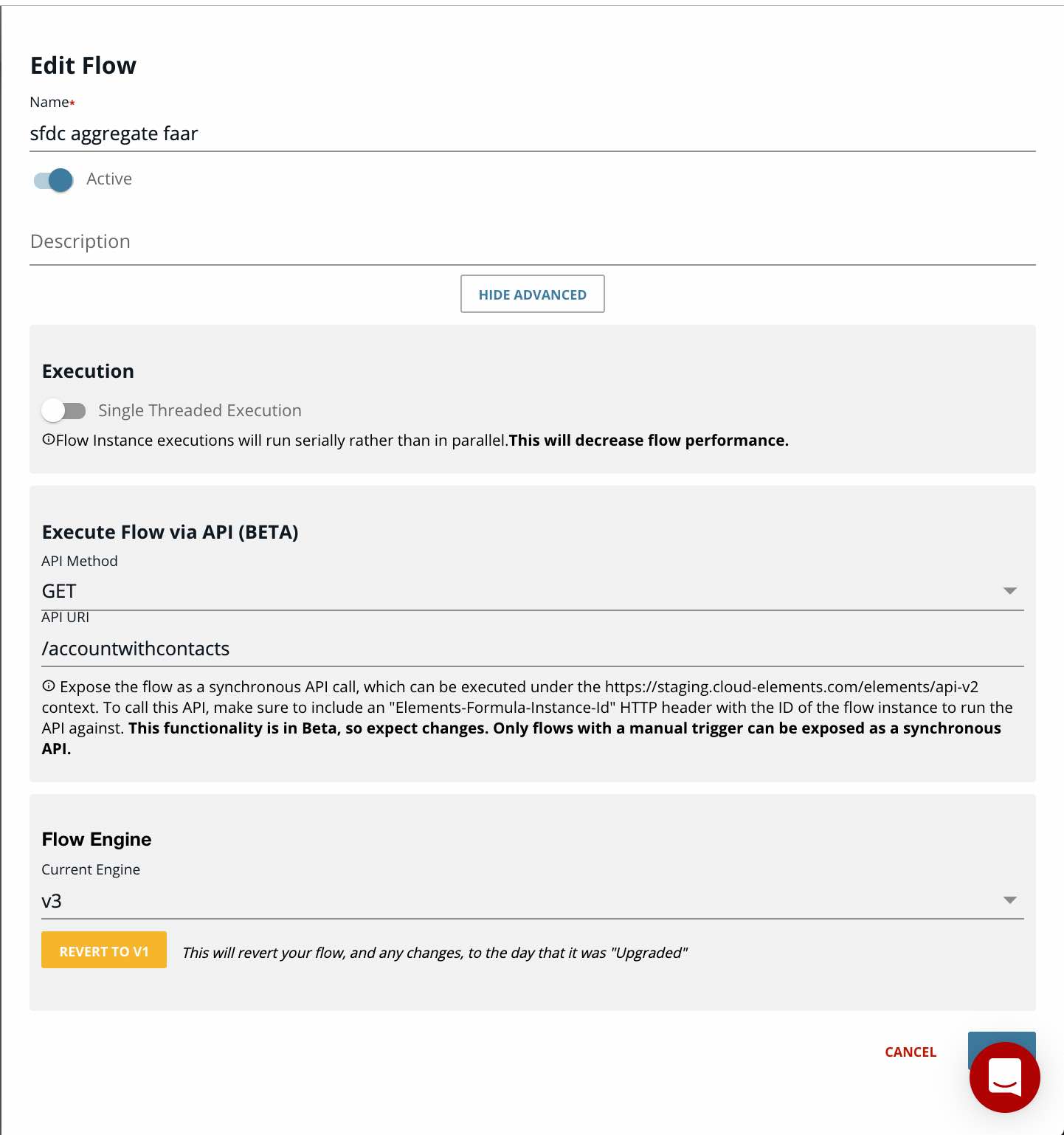
- Add a Connector Instance variable so we can attach the Salesforce connector to our flow.
Add Steps
My flow is shown below:
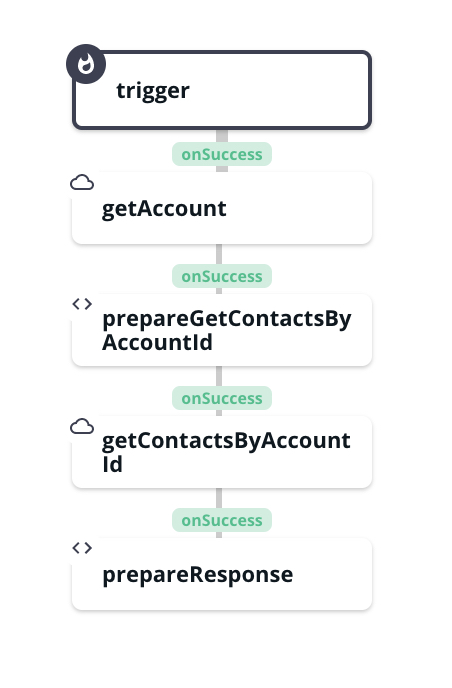
The first step is a Connector API Request step called getAccount to retrieve the account info based on the passed in account id
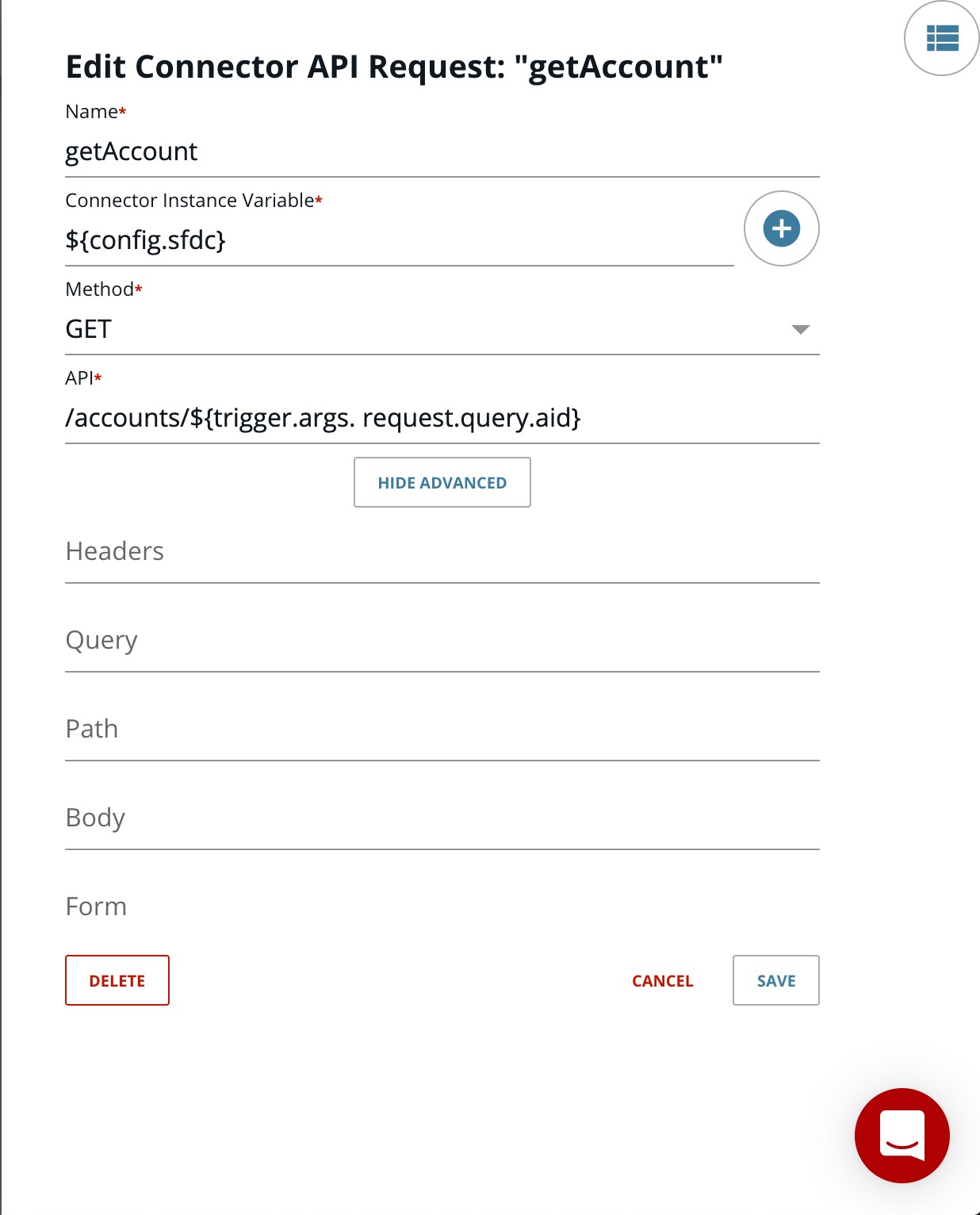
Note that we should first check to make sure that an Account ID is passed in as a query parameter as you can see that we are using that as part of the API URL but I am skipping that for this example to keep the flow and blog post more succinct
The next step is a JS Script step called prepareGetContactsByAccountId. It creates the Salesforce contact query API URL required for the next step as follows:
let where = "where=AccountId='"+trigger.args. request.query.aid+"'"; let url = "/contacts?"+where; done({url:url});
It’s basically retrieving all contacts that have an Account ID equal to the Account ID passed in the API request.
The next step is a Connector API Request called getContactsByAccountId
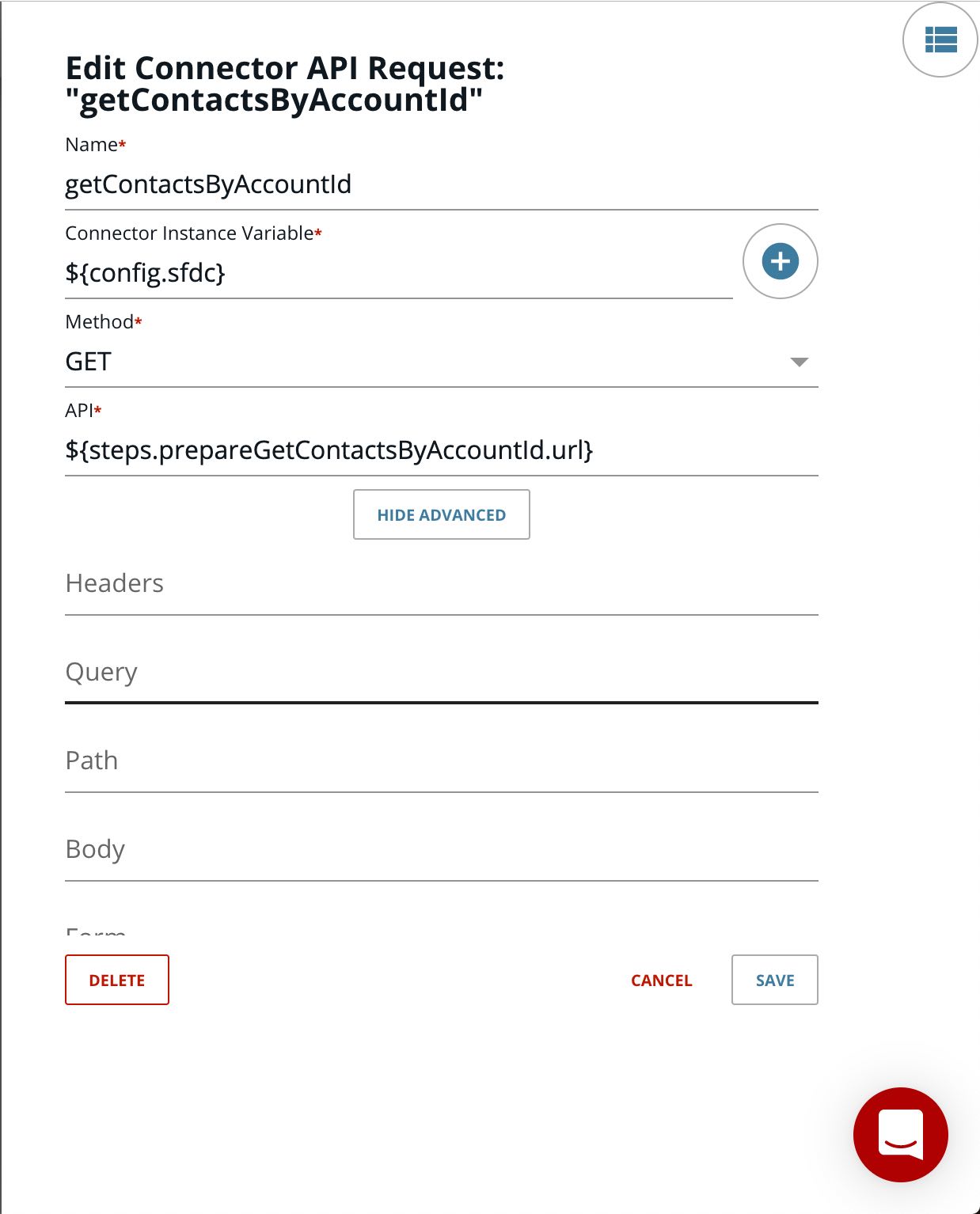
The last step is a JS Script step called prepareResponse that creates the response object described above:
done({ statusCode: 200, result: { account: steps.getAccount.response.body, contacts: steps.getContactsByAccountId.response.body } });
Call your API
I will use the following curl command to call my API:
curl -H 'Elements-Formula-Instance-Id: 417907' "https://test-xxxxxxxxxxx.apicentral.axwayamplify.com/sfdcaggregatefaar_sandbox_flow_417907-/accountwithcontacts?aid=001i000000Pscf0AAB"
Note that the above URL needs to be changed to your URL in API Central
The response is:
{ "account": { "LastModifiedDate": "2015-05-05T12:54:49.000+0000", "Ownership": "Public", "lb_sftest__UpsellOpportunity__c": "Maybe", "BillingCity": "Burlington", "lb_sftest__SLA__c": "Silver", "Rating": "Warm", "Website": "www.burlington.com", "LastReferencedDate": "2019-12-11T19:54:07.000+0000", "NumberOfEmployees": 9000, "Name": "Burlington Textiles Corp of America", "Industry": "Apparel", "BillingAddress": { "country": "USA", "city": "Burlington", "street": "525 S. Lexington Ave\r\nBurlington\r\nNC\r\n27215\r\nUSA", "postalCode": "27215", "state": "NC" }, "CreatedById": "005i0000001iEVhAAM", "OwnerId": "005i0000001iEVhAAM", "TickerSymbol": "BTXT", "Phone": "(336) 222-7000", "BillingPostalCode": "27215", "PhotoUrl": "/services/images/photo/001i000000Pscf0AAB", "lb_sftest__SLAExpirationDate__c": "2012-10-26", "IsDeleted": false, "lb_sftest__NumberofLocations__c": 6, "lb_sftest__SLASerialNumber__c": "5367", "LastViewedDate": "2019-12-11T19:54:07.000+0000", "Sic": "546732", "AccountNumber": "CD656092", "SystemModstamp": "2015-05-05T12:54:49.000+0000", "Type": "Customer - Direct", "BillingCountry": "USA", "BillingStreet": "525 S. Lexington Ave\r\nBurlington\r\nNC\r\n27215\r\nUSA", "CreatedDate": "2013-09-30T21:36:31.000+0000", "attributes": { "type": "Account", "url": "/services/data/v47.0/sobjects/Account/001i000000Pscf0AAB" }, "Id": "001i000000Pscf0AAB", "Fax": "(336) 222-8000", "LastModifiedById": "005i0000001iEVhAAM", "BillingState": "NC", "AnnualRevenue": 350000000 }, "contacts": [ { "LastModifiedDate": "2019-12-05T17:36:02.000+0000", "IsDeleted": false, "AccountId": "001i000000Pscf0AAB", "Email": "lbrenman99@hotmail.com", "IsEmailBounced": false, "Description": "Contact for Leor Brenman", "FirstName": "John", "LastViewedDate": "2019-12-11T18:53:34.000+0000", "Title": "Purchasing Agent", "LastReferencedDate": "2019-12-11T18:53:34.000+0000", "MobilePhone": "+16176428274", "Name": "John Doe", "SystemModstamp": "2019-12-05T17:36:02.000+0000", "CreatedById": "005i0000001iEVhAAM", "OwnerId": "005i0000001iEVhAAM", "Phone": "+1-(617) 642-8274", "CreatedDate": "2019-06-05T18:50:47.000+0000", "PhotoUrl": "/services/images/photo/0030H000058R5yTQAS", "Id": "0030H000058R5yTQAS", "LastName": "Doe", "LastModifiedById": "005i0000001iEVhAAM" }, { "LastModifiedDate": "2019-12-05T19:32:08.000+0000", "AccountId": "001i000000Pscf0AAB", "Email": "griffin-lab@axway.com", "Description": "Contact for Darren M", "MailingCountry": "USA", "LastReferencedDate": "2019-12-11T19:54:06.000+0000", "MobilePhone": "+16175551212", "MailingState": "NC", "Name": "Jim Doe", "CreatedById": "005i0000001iEVhAAM", "OwnerId": "005i0000001iEVhAAM", "Phone": "+16175551212", "MailingPostalCode": "27215", "PhotoUrl": "/services/images/photo/0030H00005GbzcHQAR", "IsDeleted": false, "IsEmailBounced": false, "FirstName": "Jim", "LastViewedDate": "2019-12-11T19:54:06.000+0000", "MailingCity": "Burlington", "MailingAddress": { "country": "USA", "city": "Burlington", "street": "525 S. Lexington Ave\r\nBurlington\r\nNC\r\n27215\r\nUSA", "postalCode": "27215", "state": "NC" }, "SystemModstamp": "2019-12-05T19:32:08.000+0000", "CreatedDate": "2019-12-05T17:35:25.000+0000", "Id": "0030H00005GbzcHQAR", "LastName": "Doe", "LastModifiedById": "005i0000001iEVhAAM", "MailingStreet": "525 S. Lexington Ave\r\nBurlington\r\nNC\r\n27215\r\nUSA" }, { "LastModifiedDate": "2013-09-30T21:36:31.000+0000", "AccountId": "001i000000Pscf0AAB", "Email": "jrogers@burlington.com", "MailingCountry": "USA", "Salutation": "Mr.", "MailingState": "NC", "Name": "Jack Rogers", "CreatedById": "005i0000001iEVhAAM", "OwnerId": "005i0000001iEVhAAM", "Phone": "(336) 222-7000", "MailingPostalCode": "27215", "PhotoUrl": "/services/images/photo/003i000000M33B5AAJ", "IsDeleted": false, "IsEmailBounced": false, "FirstName": "Jack", "Title": "VP, Facilities", "MailingCity": "Burlington", "MailingAddress": { "country": "USA", "city": "Burlington", "street": "525 S. Lexington Ave", "postalCode": "27215", "state": "NC" }, "SystemModstamp": "2013-09-30T21:36:31.000+0000", "LeadSource": "Web", "CreatedDate": "2013-09-30T21:36:31.000+0000", "Id": "003i000000M33B5AAJ", "LastName": "Rogers", "Fax": "(336) 222-8000", "LastModifiedById": "005i0000001iEVhAAM", "MailingStreet": "525 S. Lexington Ave" } ] }
Summary
In this blog post, we saw how we can use Integration Builder’s Flow as a Resource (FaaR) feature to create a complex, aggregate API.
Learn how you can create a reusable code with Axway’s Integration Builder.
Follow us on social