Axway’s API Builder makes it easy to make Axway Amplify platform API calls from within a flow. This can be useful for implementing Unified Catalog custom API Subscription flows and Amplify Integrations. In this blog post, we’ll create a simple API Builder Hello World flow that will make an Amplify platform API call to illustrate the basic steps.
In order to make Amplify Platform API calls you will need a clientId and clientSecret as described here.
Once you have a clientId and clientSecret the steps will be:
- Create an API Builder project
- Get the swagger definition for the Amplify platform API and place it in the API Builder project
/swagger
folder - Authenticate the Amplify API by entering the credentials (e.g. clientId and clientSecret) in the swagger credentials file in API Builder
- Use the resulting Amplify platform API swagger flow node in a flow to make an API call
Create an API Builder Project
Follow the API Builder Getting Started Guide to create an API Builder project. Make sure the project runs using npm start
(as described in the guide).
Create the Amplify platform API Swagger File
Axway Platform APIs, including Unified Catalog and Central can be found here. For this example, we’ll look at the Amplify Unified Catalog API’s GET /catalogItems
service to retrieve a list of API’s in the Unified Catalog in my organization.
My list of API’s in the Unified Catalog is shown here for illustration purposes:
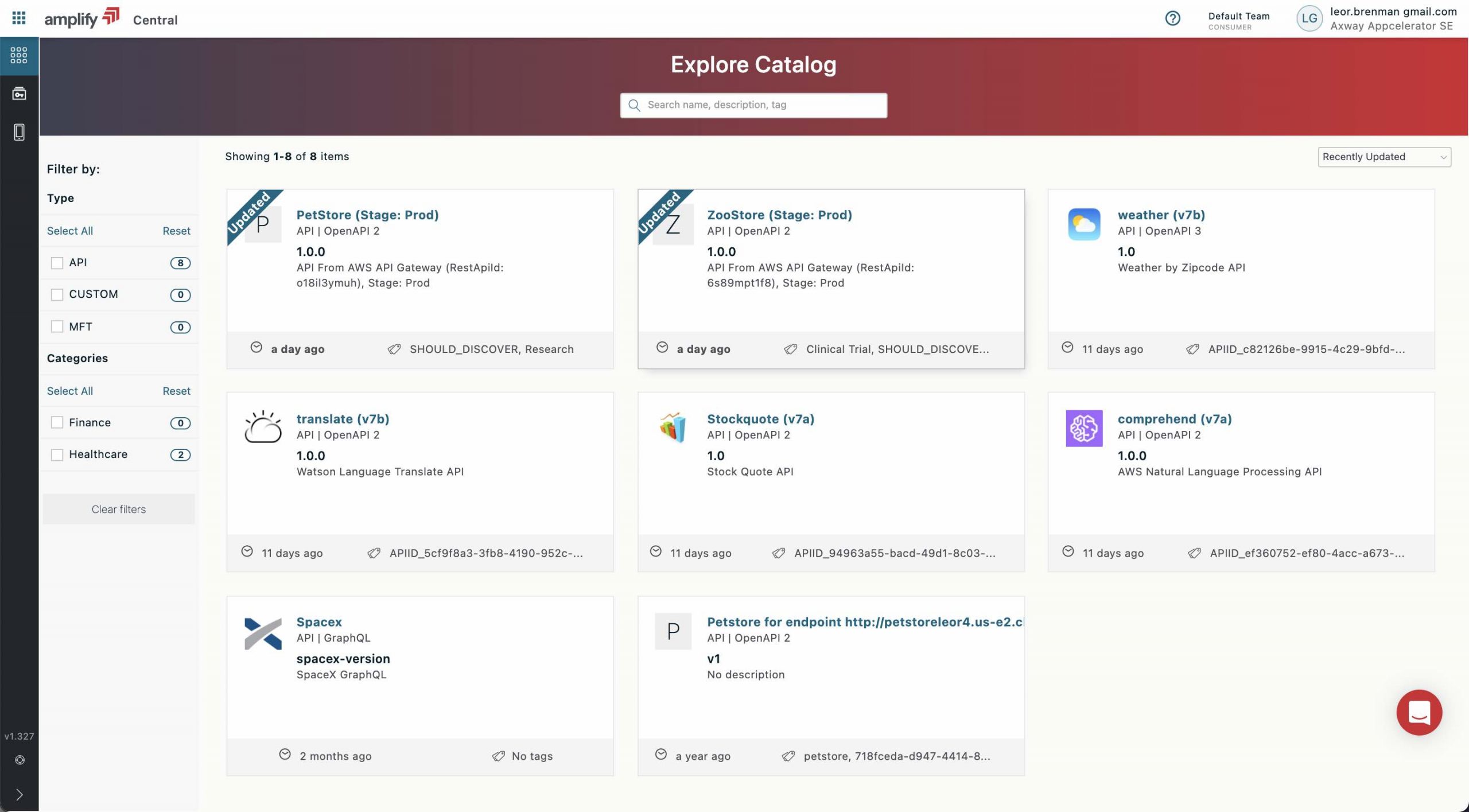
On the Amplify Unified Catalog API page click the swagger.json link to get to the swagger.
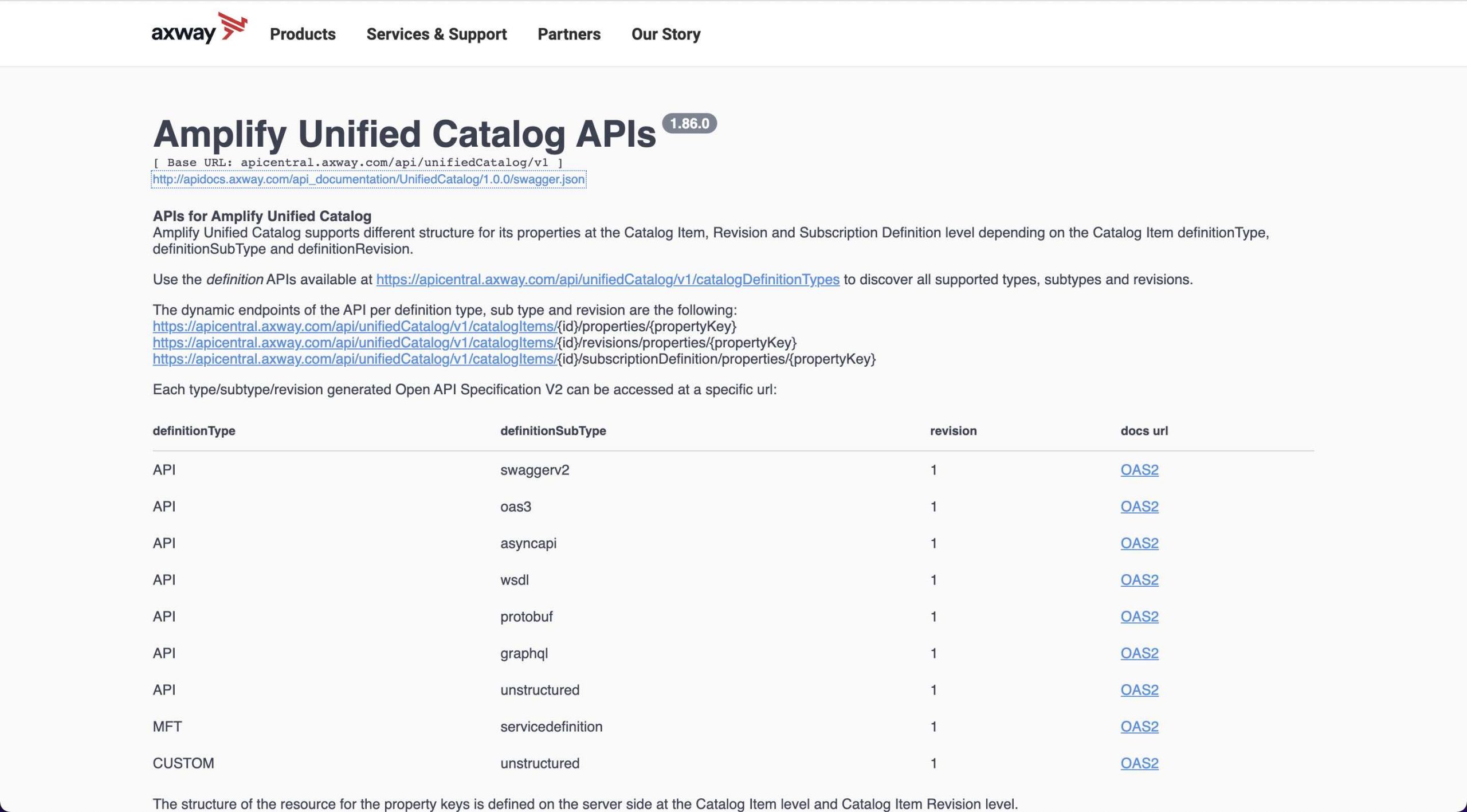
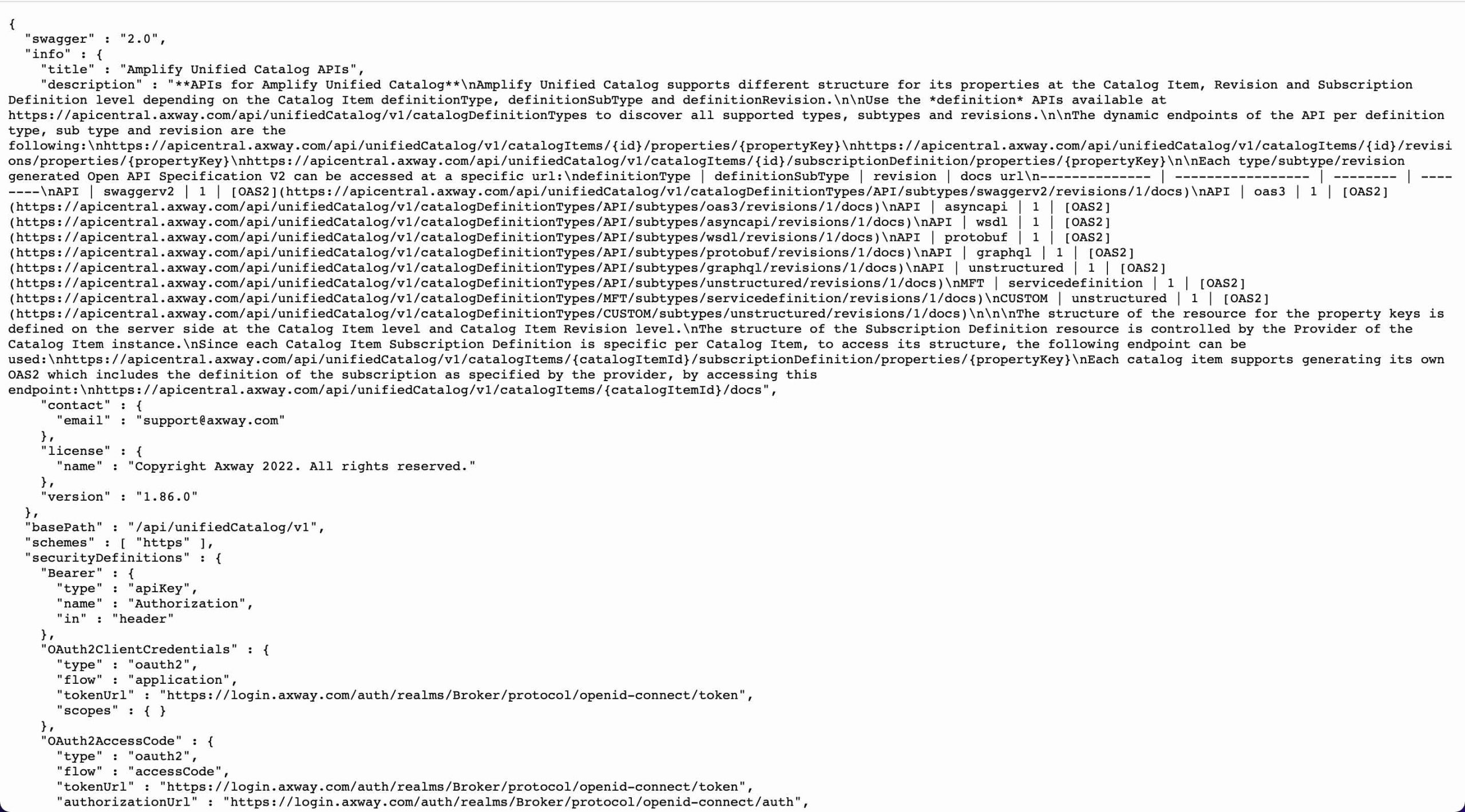
Note that the swagger corresponds the US region. Use this swagger for the EU region.
Copy the swagger JSON contents to a file, e.g. ucswagger.json
and copy the swagger file to the API Builder /swagger
folder and restart your API Builder project (npm start
).
Authenticate the Amplify API
When you start your project and launch the API Builder console at http://localhost:8080/console
you will notice a red badge in the Credentials tab indicating that you need to authenticate the Amplify Unified Catalog API.
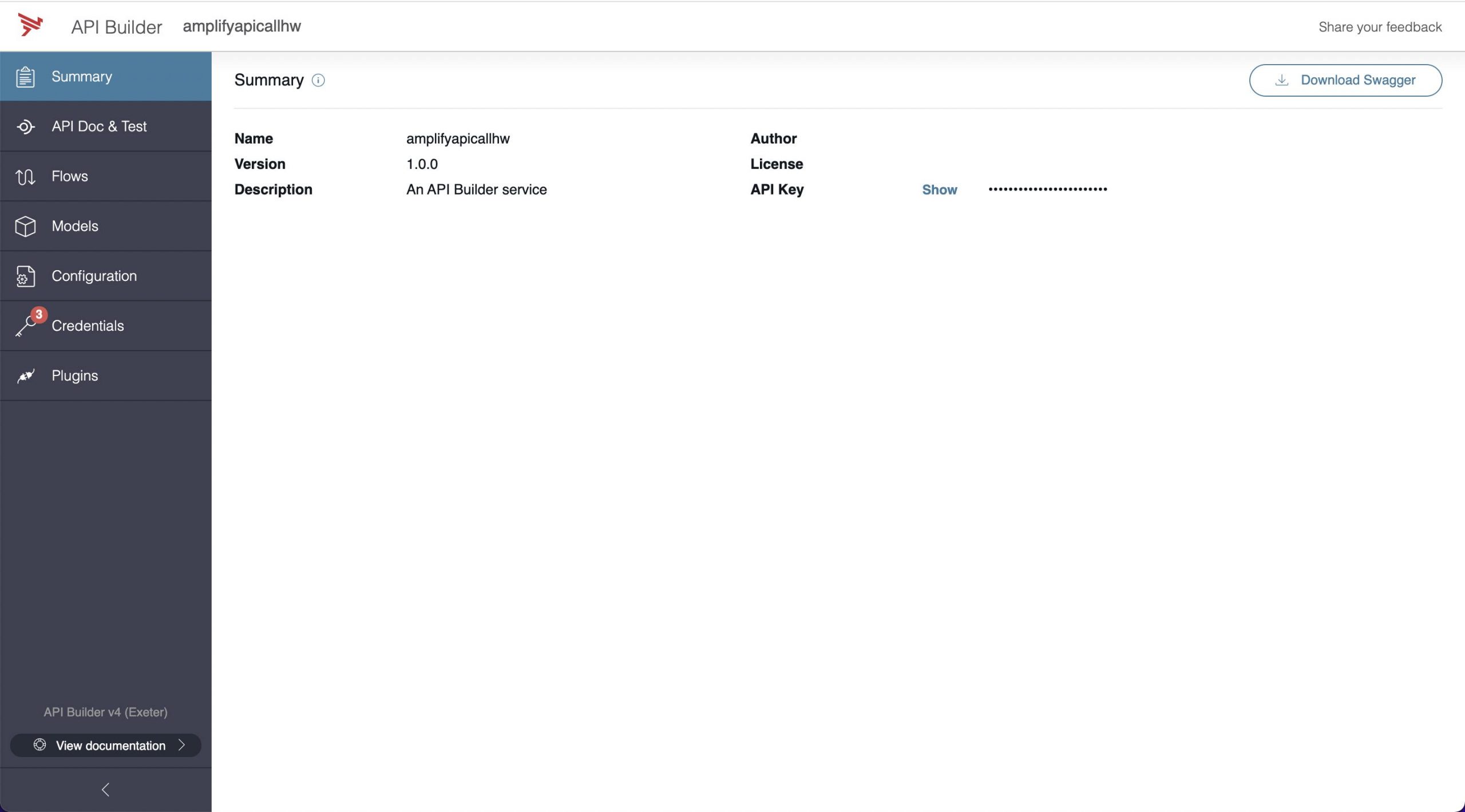
Click on the Configuration tab and then click on the swagger configuration file (ucswagger.default.js
, in my case).
We are going to use OAuth 2.0 client credentials (client password) for authentication. You can see how API Builder supports this here.
Modify the Authorization portion of the configuration file (above) to the following and click save.
authorization: { credentials: { 'Amplify Unified Catalog APIs OAuth2ClientCredentials_clientCredentials': { type: 'oauth2', flow: 'clientCredentials', token_url: 'https://login.axway.com/auth/realms/Broker/protocol/openid-connect/token', scope: '', client_id: process.env.clientId, client_secret: process.env.clientSecret, access_token: null } } }
Note that I removed the other authorization schemes that don’t apply to me and I needed to add the client_secret property.
Note that I am referencing the clientId and clientSecret as environment variables. You can add your environment variable to the /conf/.env
file or set the environment variables when you start your API Builder project as described here
Modify the host if required as follows:
You will see that the red badge on the Configurations tab will turn orange and then disappear as the access_token is retrieved.
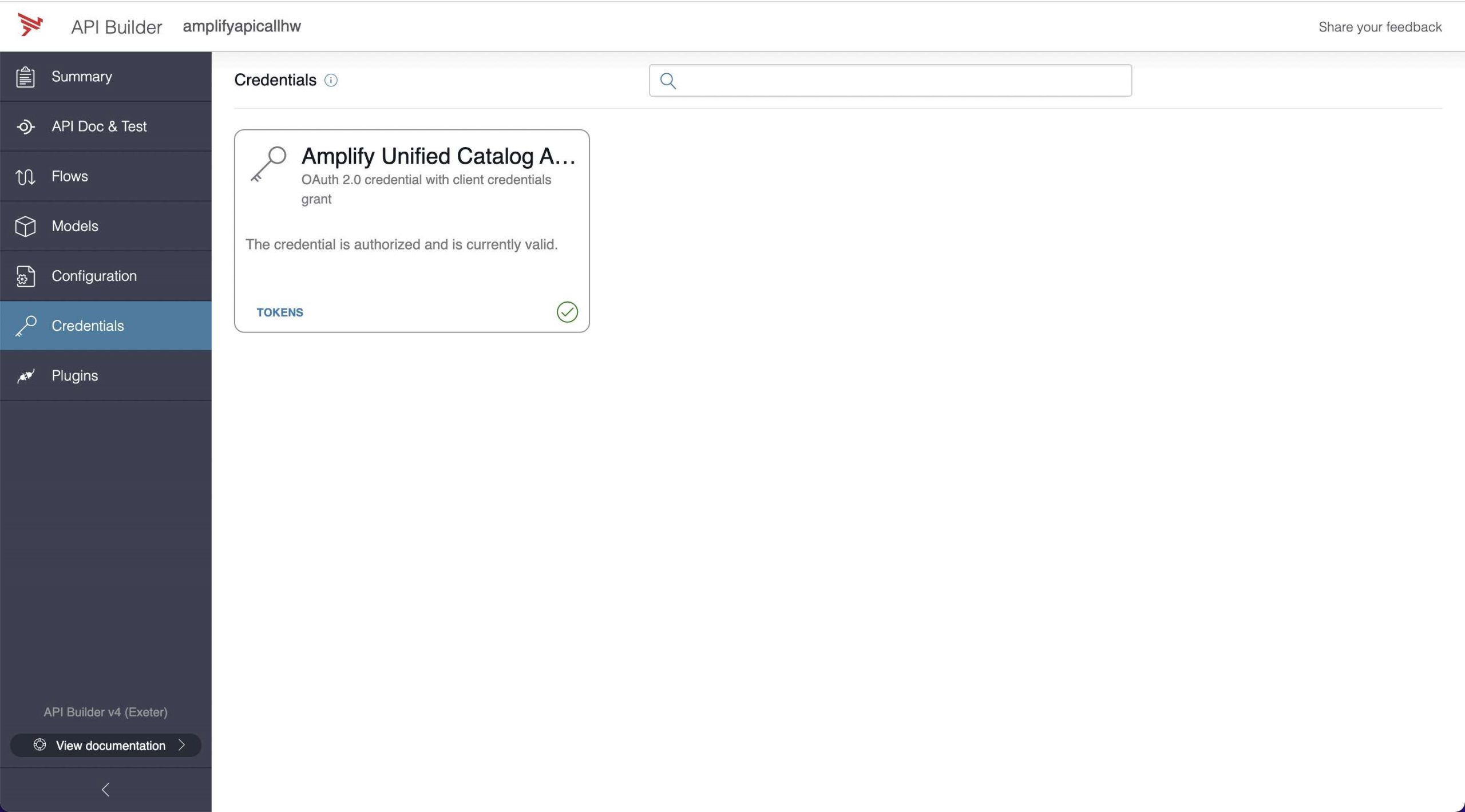
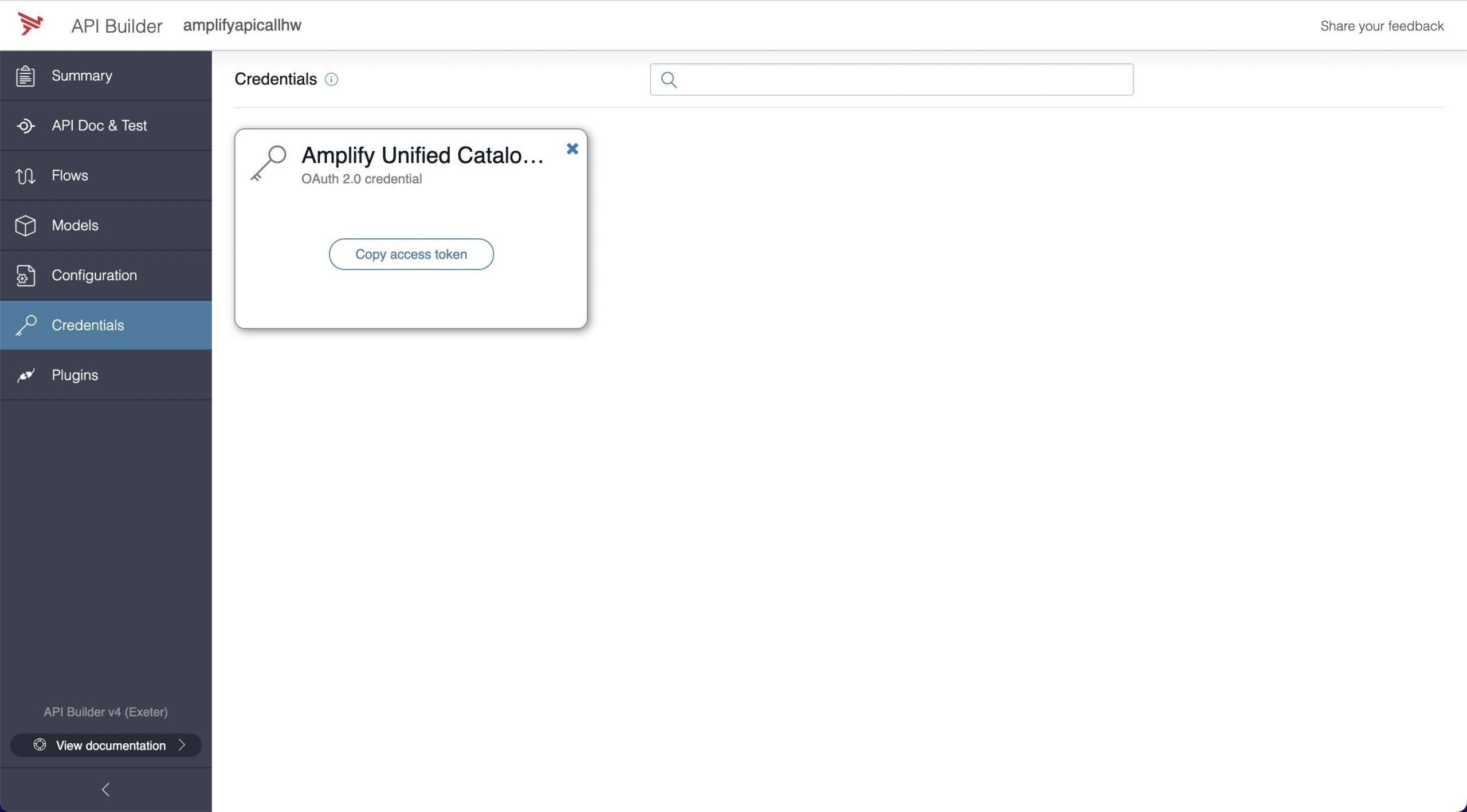
You can click on the Copy access token button and paste you token in a text editor to review but this is not necessary.
Test The Unified Catalog API
Click on the sample Greeting API in the API Doc & Test tab.
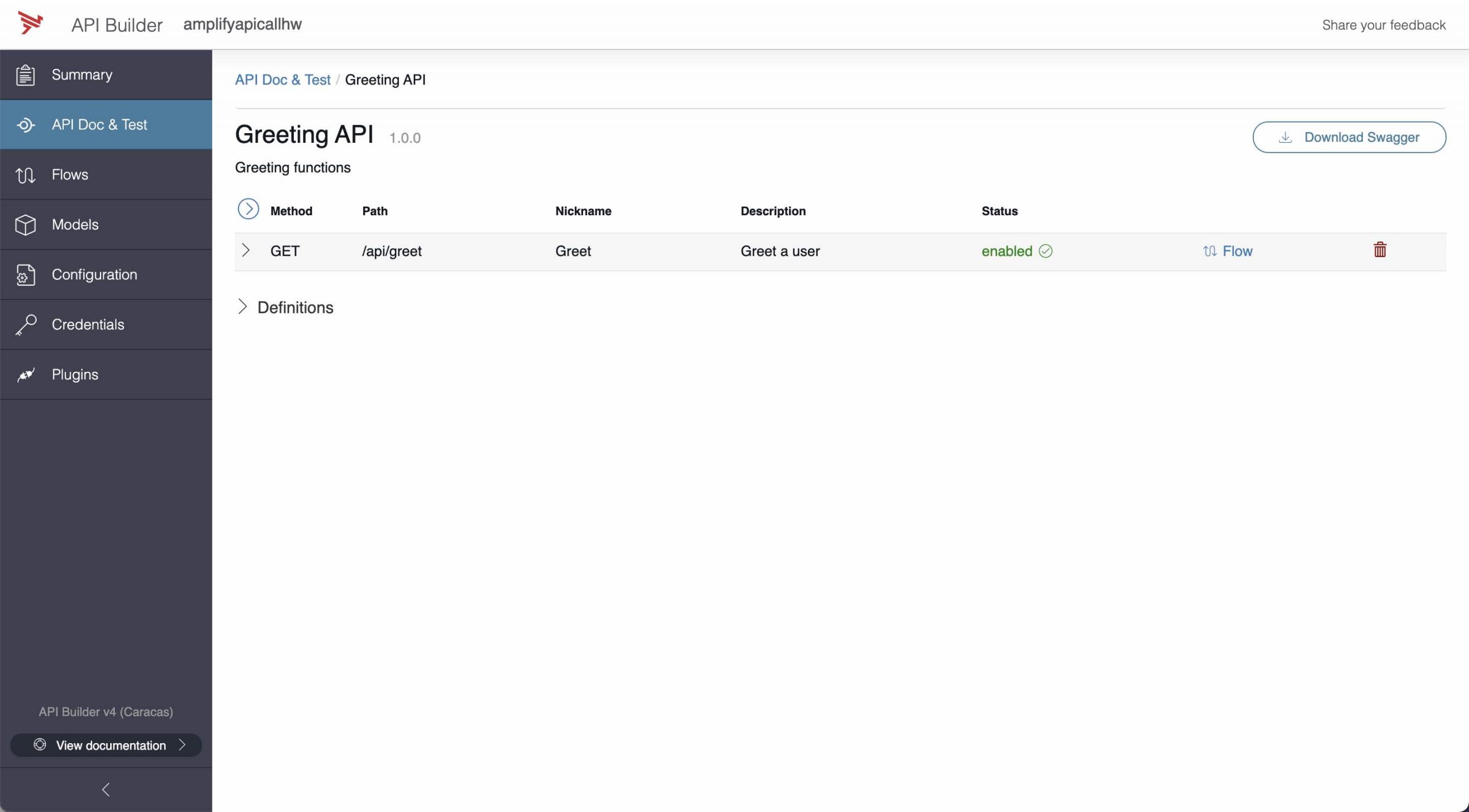
Click on the Flow hyperlink to edit the flow.
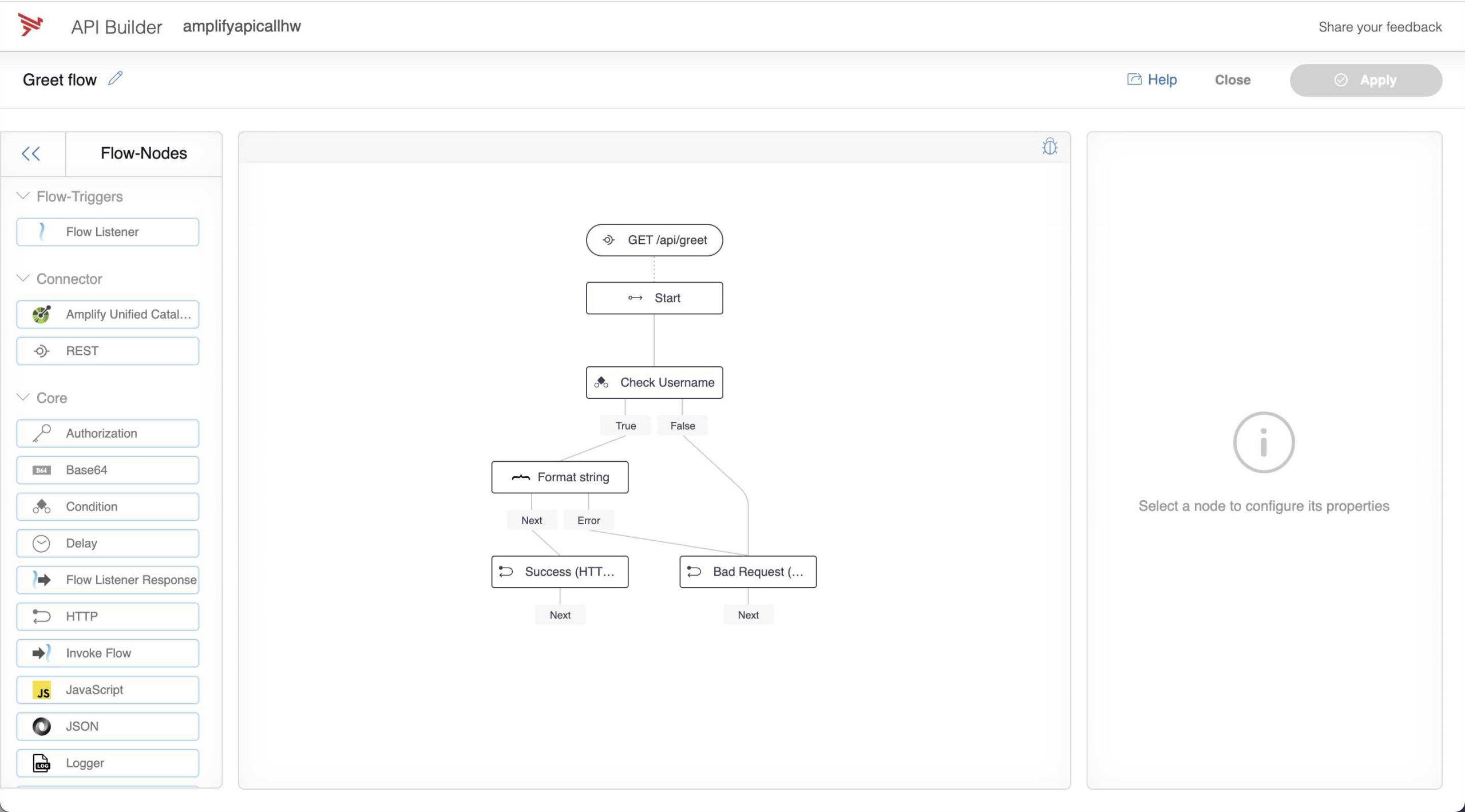
Note the Amplify Unified Catalog APIs connector in the Flow-Nodes Connector section.
Drag the Amplify Unified Catalog APIs connector onto the canvas and drag a connection from the Start node to the Amplify Unified Catalog APIs connector node.
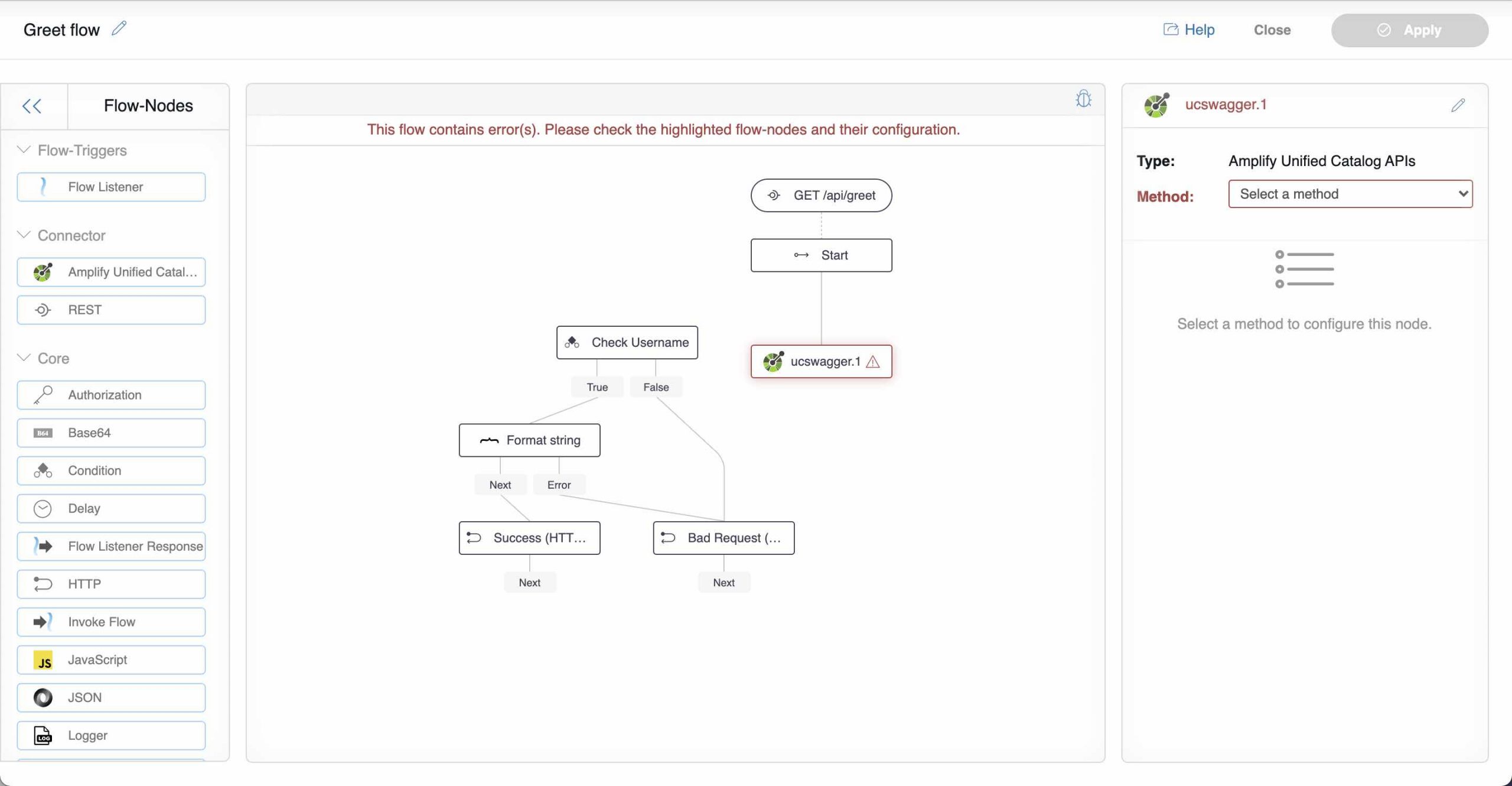
Name your Amplify Unified Catalog APIs connector node (e.g. Get Catalog Items) and select GET_catalogItems in the Method picker.
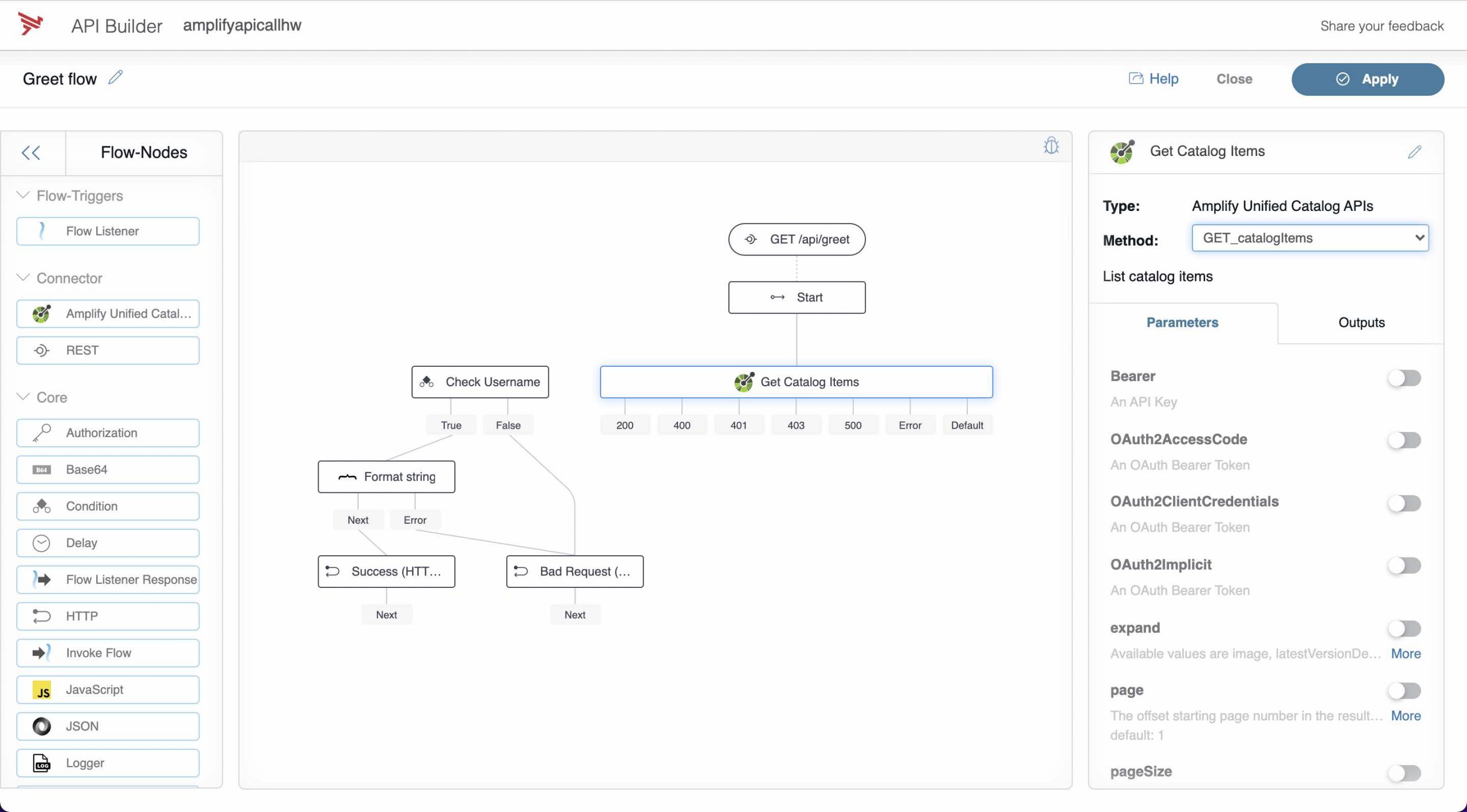
Toggle the OAuth2ClientCredentials property and select the available credential.
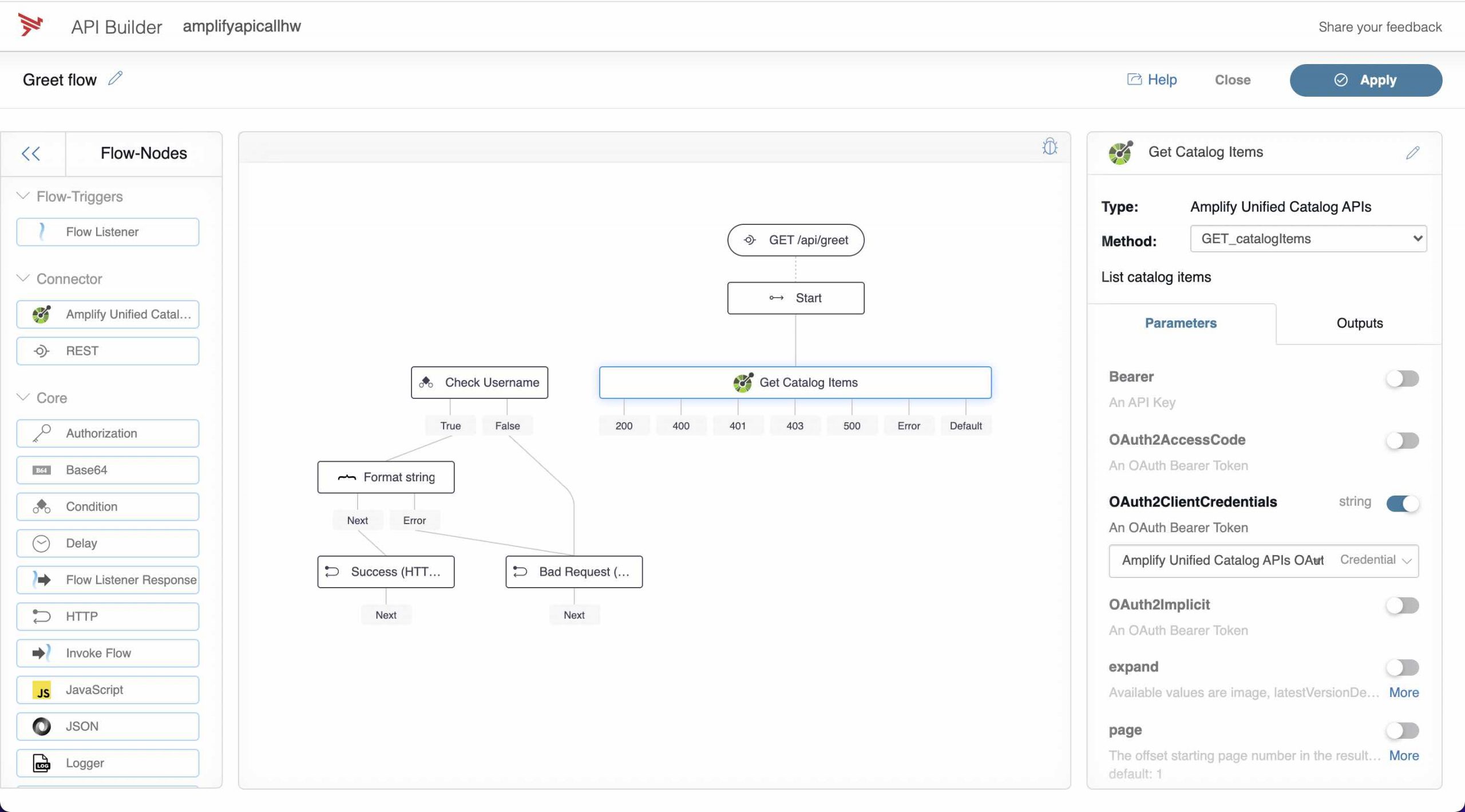
Drag a connection from the 200 output of the Get Catalog Items connector node to the Success (HTTP 200) node and remove the connection from this node. Set the body to $.response as this is the output of the Get Catalog Items connector node.
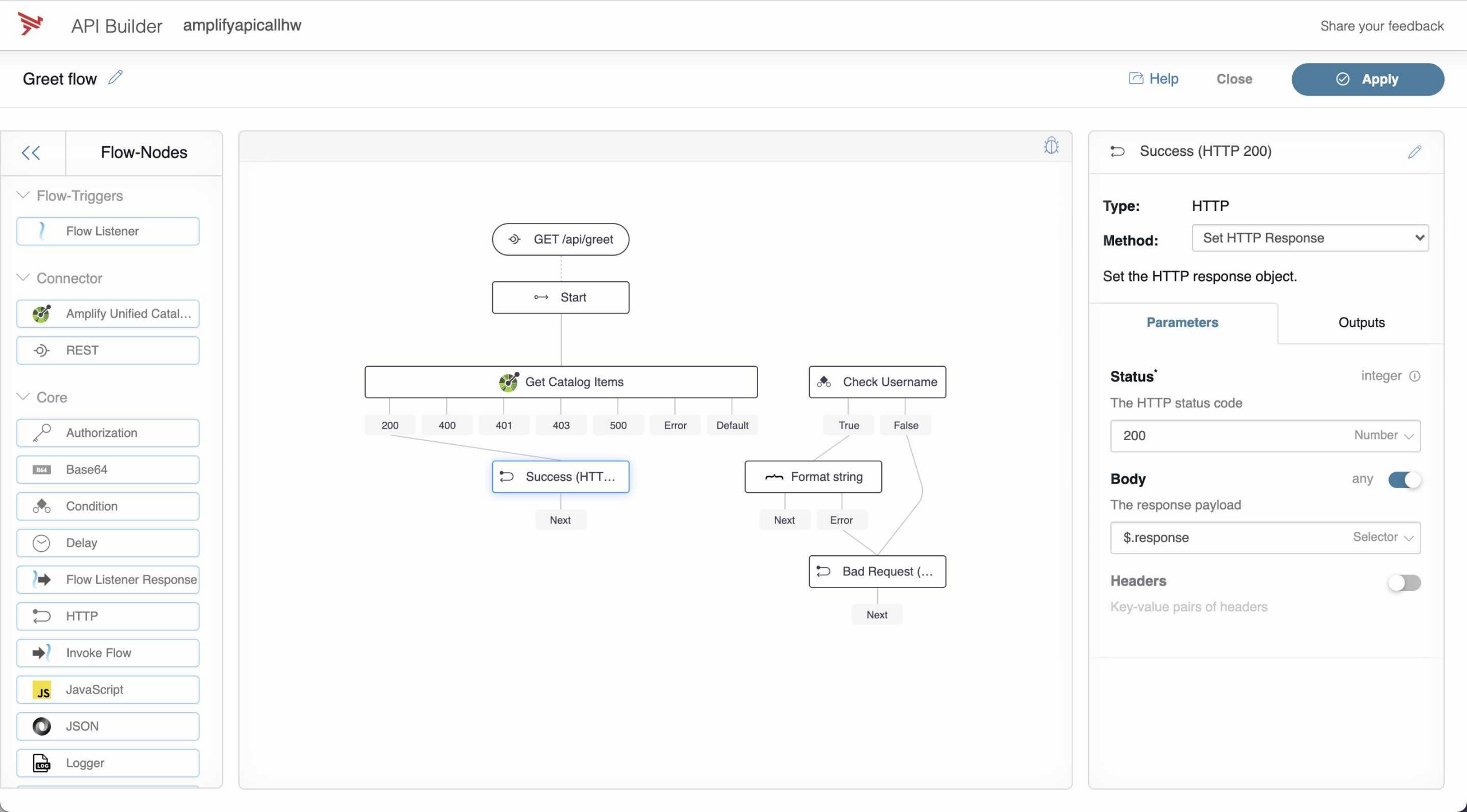
Click Apply and close the flow.
Go back to the API Doc & Test tab and click on the Greeting API, expand it, enter any string for the username and click the Execute button and see the list of Catalog Items.
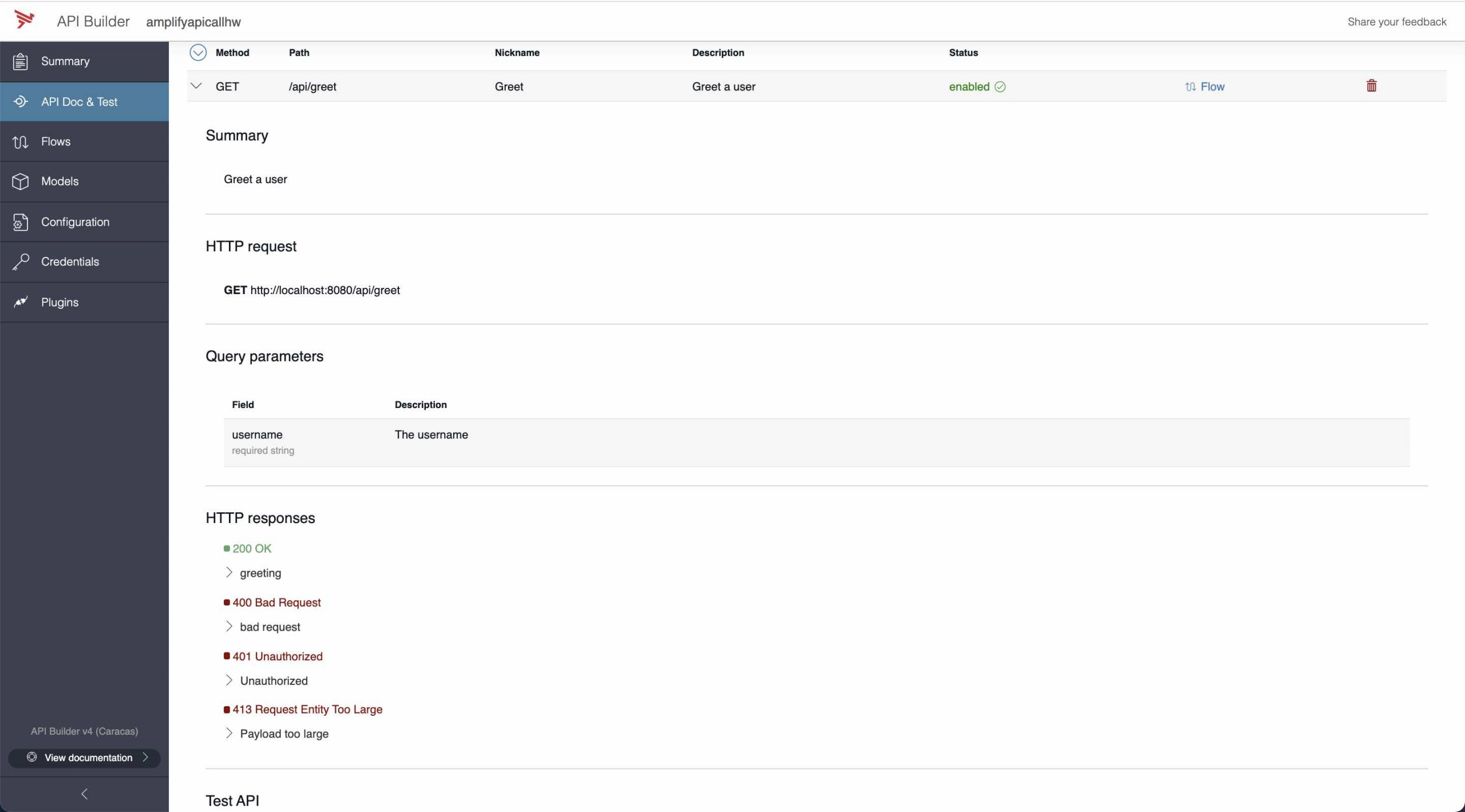
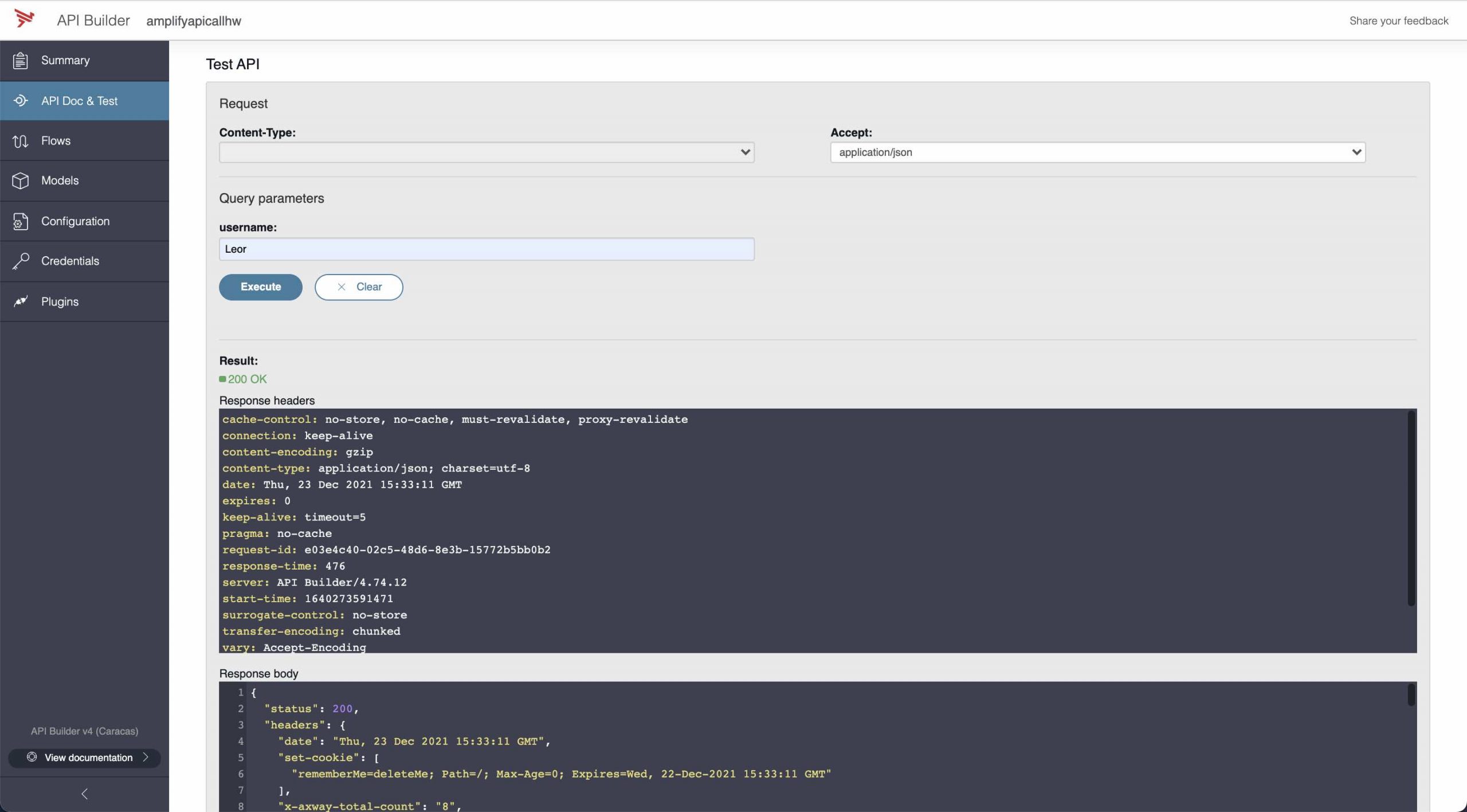
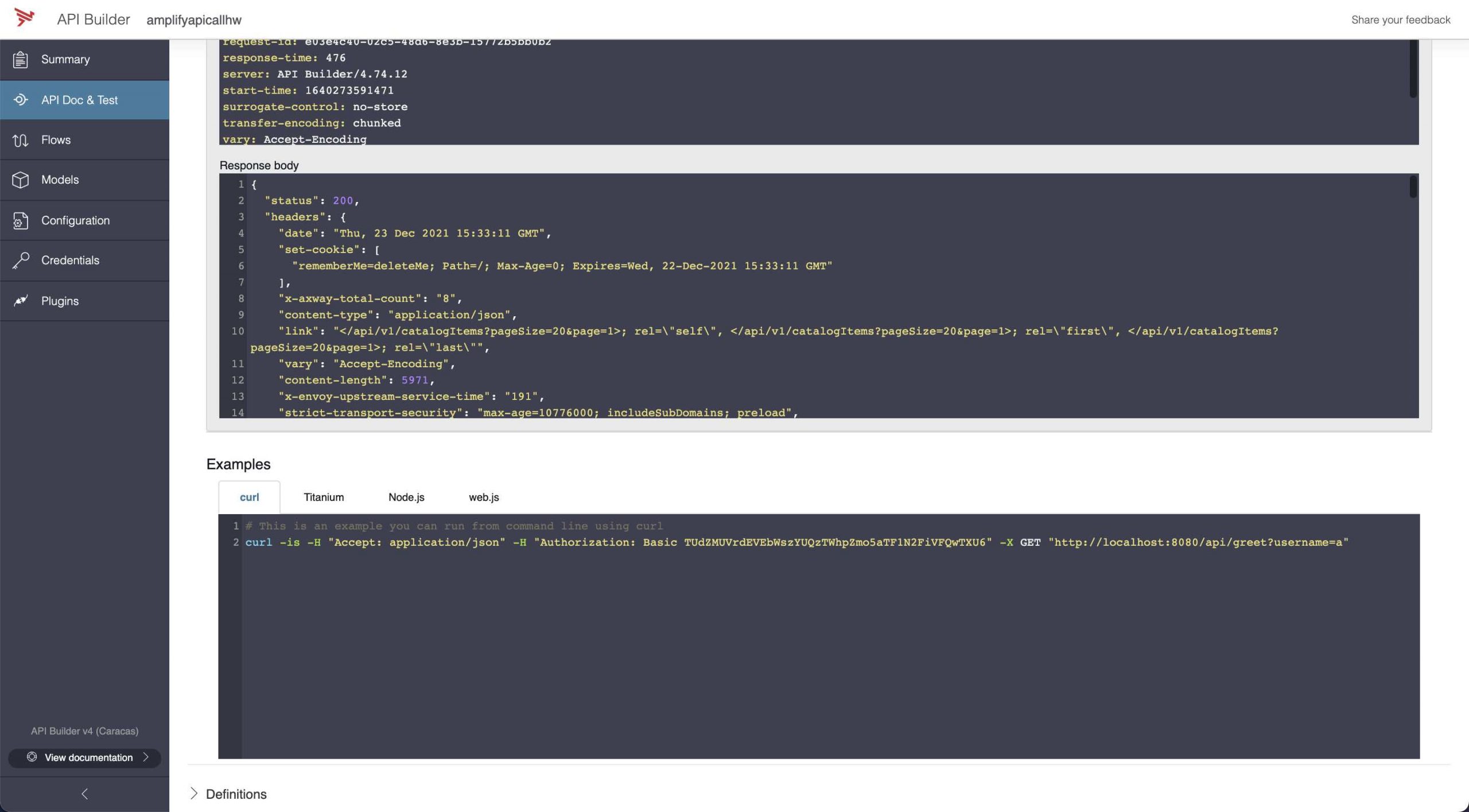
The results should look similar to the following:
{
"status": 200,
"headers": {
"date": "Thu, 23 Dec 2021 15:33:11 GMT",
"set-cookie": [
"rememberMe=deleteMe; Path=/; Max-Age=0; Expires=Wed, 22-Dec-2021 15:33:11 GMT"
],
"x-axway-total-count": "8",
"content-type": "application/json",
"link": "</api/v1/catalogItems?pageSize=20&page=1>; rel=\"self\", </api/v1/catalogItems?pageSize=20&page=1>; rel=\"first\", </api/v1/catalogItems?pageSize=20&page=1>; rel=\"last\"",
"vary": "Accept-Encoding",
"content-length": 5971,
"x-envoy-upstream-service-time": "191",
"strict-transport-security": "max-age=10776000; includeSubDomains; preload",
"content-security-policy": "frame-ancestors none",
"x-content-type-options": "nosniff",
"x-xss-protection": "1; mode=block",
"server": "envoy"
},
"data": [
{
"id": "8a2e8a147c9a7cae017c9dcf61d702f3",
"owningTeamId": "e4ec6c1a69fd0b8e016a22c82fc9170c",
"definitionType": "API",
"definitionSubType": "graphql",
"definitionRevision": 1,
"name": "Spacex",
"description": "SpaceX GraphQL",
"tags": [],
"metadata": {
"createTimestamp": "2021-10-20T13:06:26.394+0000",
"createUserId": "789f8247a10c466f36894f0f3cfdce19",
"modifyTimestamp": "2021-10-20T13:06:26.518+0000",
"modifyUserId": "789f8247a10c466f36894f0f3cfdce19"
},
"visibility": "PUBLIC",
"state": "PUBLISHED",
"access": "admin",
"availableRevisions": [
1
],
"latestVersion": "spacex-version",
"totalSubscriptions": 0
},
{
"id": "8a2e8be87d5b2072017daab63fed7631",
"owningTeamId": "e4ec6c1a69fd0b8e016a22c82fc9170c",
"definitionType": "API",
"definitionSubType": "swaggerv2",
"definitionRevision": 1,
"name": "comprehend (v7a)",
"description": "AWS Natural Language Processing API",
"tags": [
"APIID_ef360752-ef80-4acc-a673-ef92f3d62411"
],
"metadata": {
"createTimestamp": "2021-12-11T18:16:50.414+0000",
"createUserId": "DOSA_ced1f1cf6c8048e89eb591065a6c7813",
"modifyTimestamp": "2021-12-11T18:16:50.454+0000",
"modifyUserId": "DOSA_ced1f1cf6c8048e89eb591065a6c7813"
},
"visibility": "RESTRICTED",
"state": "PUBLISHED",
"access": "admin",
"availableRevisions": [
1
],
"latestVersion": "1.0.0",
"totalSubscriptions": 0
},
.
.
.
{
"id": "e4f9db18756c215601756c847e0e7706",
"owningTeamId": "e4ec6c1a69fd0b8e016a22c82fc9170c",
"definitionType": "API",
"definitionSubType": "swaggerv2",
"definitionRevision": 1,
"name": "Petstore for endpoint http://petstoreleor4.us-e2.cloudhub.io",
"description": "",
"tags": [
"petstore",
"718fceda-d947-4414-84d5-7ccbe580245b",
"oas2",
"Self"
],
"metadata": {
"createTimestamp": "2020-10-28T00:03:43.759+0000",
"modifyTimestamp": "2020-10-28T00:03:43.759+0000"
},
"visibility": "RESTRICTED",
"state": "PUBLISHED",
"access": "admin",
"availableRevisions": [
1
],
"latestVersion": "v1",
"totalSubscriptions": 0
}
]
}
The result is the list of Unified Catalog items I have in my Organization.
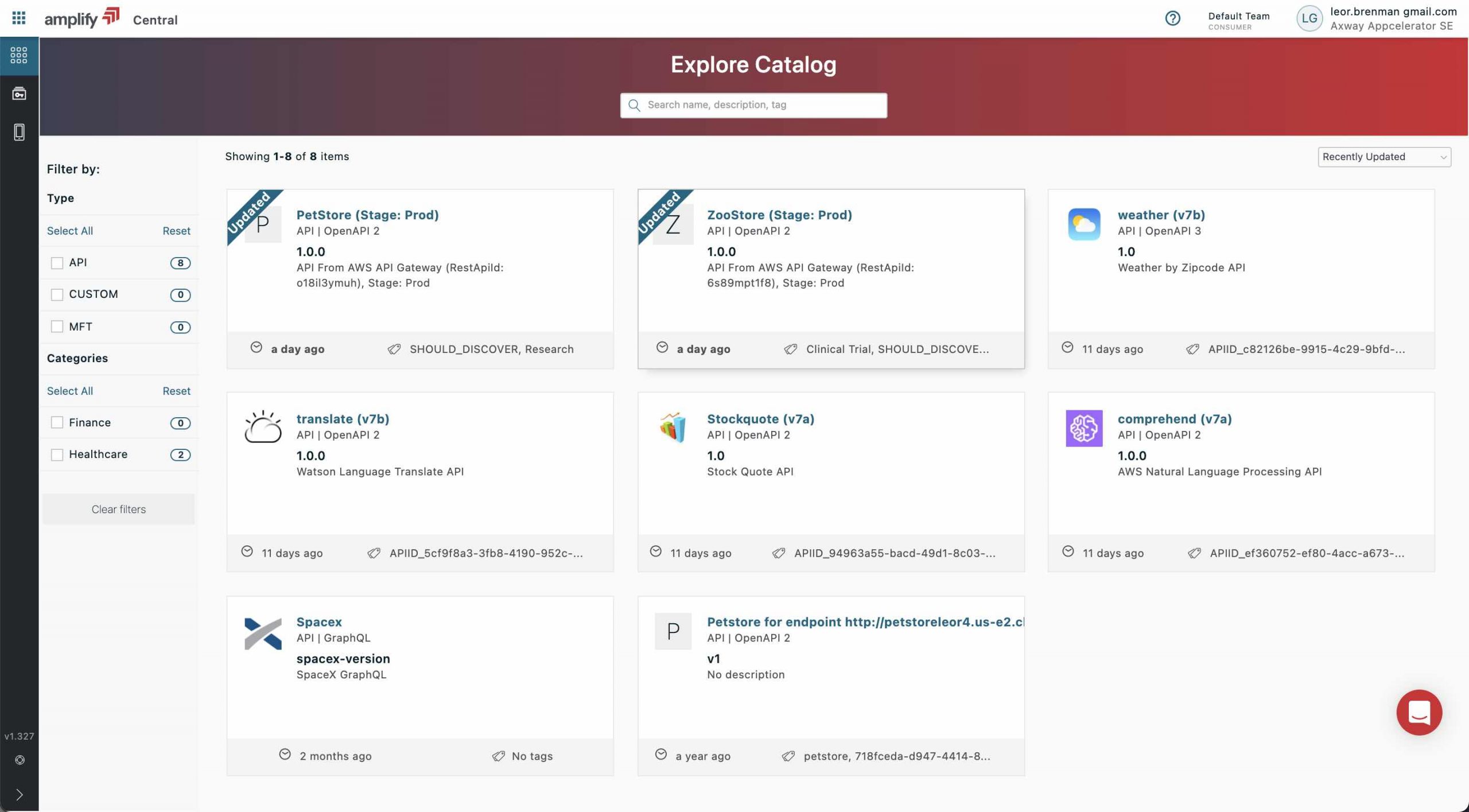
Summary
In this blog post we saw how to make Axway Platform API calls using a Service Account in API Builder Flows. We demonstrated how API Builder can (1) leverage the Platform API swagger definition, (2) manage the authorization and (3) provide a no code/low code graphical API flow design in a seamless fashion reducing the burden on the developer.
The API Builder project can be found here.
Follow us on social