Axway’s API Builder provides several means of creating APIs and Microservices, including Data Connectors for connecting to databases and a Swagger flow-node and a Rest flow-node for connecting to backend resources in a flow. However, this means that either a connector must be available for the service you want to expose or the service must expose APIs.
What do you do if the service doesn’t expose REST APIs and there is no existing API Builder Data Connector for this service? If the service has a Javascript SDK, then API Builder’s Custom API feature can be used to build your APIs. AWS Comprehend natural language processing (NLP) service is one such service. There are many others.
While you can usually use the SDK in your client app or website, REST API’s are more lightweight, often have better and easier to use swagger documentation and learning curve, and usually result in a smaller code footprint.
In this blog post, we’ll show how to use API Builder’s custom API feature to expose an API to AWS Comprehend’s language detection service.
The steps are as follows:
- Create an API Builder project
- Create a custom API in your API Builder project and use the AWS-SDK Javascript SDK in your custom API
- Test your API
- Publish your API
Let’s get started.
Create an API Builder Project
Follow the API Builder Getting Started Guide to create your API Builder project.
Optionally, edit the /conf/default.js file to modify your project settings settings, such as the authentication you’d like for your API and any other parameters you’d like to modify (e.g. CORS settings).
Create a Custom API in your API Builder Project
Your project contains a sample custom api in the /api folder. You can rename this or copy to create a new custom api. I called mine, detectlanguage.js
- Since we’ll be using the aws-sdk npm in our project, we need to install the aws-sdk npm into our API Builder project using the following command line:
npm i aws-sdk
- The first thing we do in our custom API is to require and initialize the service and set the region as follows:
var AWS = require('aws-sdk');
AWS.config.update({region: 'us-east-1'});
var comprehend = new AWS.Comprehend();
Note that we need to set the AWS credentials: Access key ID and Secret access key. There are several ways to set your credentials in Node.js as described here. We’ll use the environment variable method by setting the the following environment variables:
- AWS_ACCESS_KEY_ID
- AWS_SECRET_ACCESS_KEY
We’ll set these environment variables when we run our API Builder project locally and when we deploy our API Builder project docker container.
Note: Refer to this guide for information on these credentials.
- Call the detectDominantLanguage service as described here. In the above example, the input text is defined and passed into the detectDominantLanguage method. We are going to receive the text as a query parameter of our API call.
The entire custom API file, , is shown below:
var APIBuilder = require('@axway/api-builder-runtime'); var AWS = require('aws-sdk'); AWS.config.update({ region: 'us-east-1' }); var comprehend = new AWS.Comprehend(); var detectlanguage = APIBuilder.API.extend({ group: 'Comprehend', path: '/api/detectlanguage', method: 'GET', description: 'this is an api that detects the language of the text passed in the text query parameter', parameters: { text: { description: 'the text to detect the sentiment of' } }, action: function(req, resp, next) { var params = { Text: req.params.text }; comprehend.detectDominantLanguage(params, function(err, data) { if (err) { resp.response.status(400); resp.send(err); } else { resp.response.status(200); resp.send(data); } next(); }); } }); module.exports = detectlanguage;
- Run your project:
AWS_ACCESS_KEY_ID="YOUR AWS ACCESS KEY ID" AWS_SECRET_ACCESS_KEY="YOUR AWS SECRET ACCES KEY" npm start
Test Your API
Launch the console at http://localhost:8080/console and go to your API:
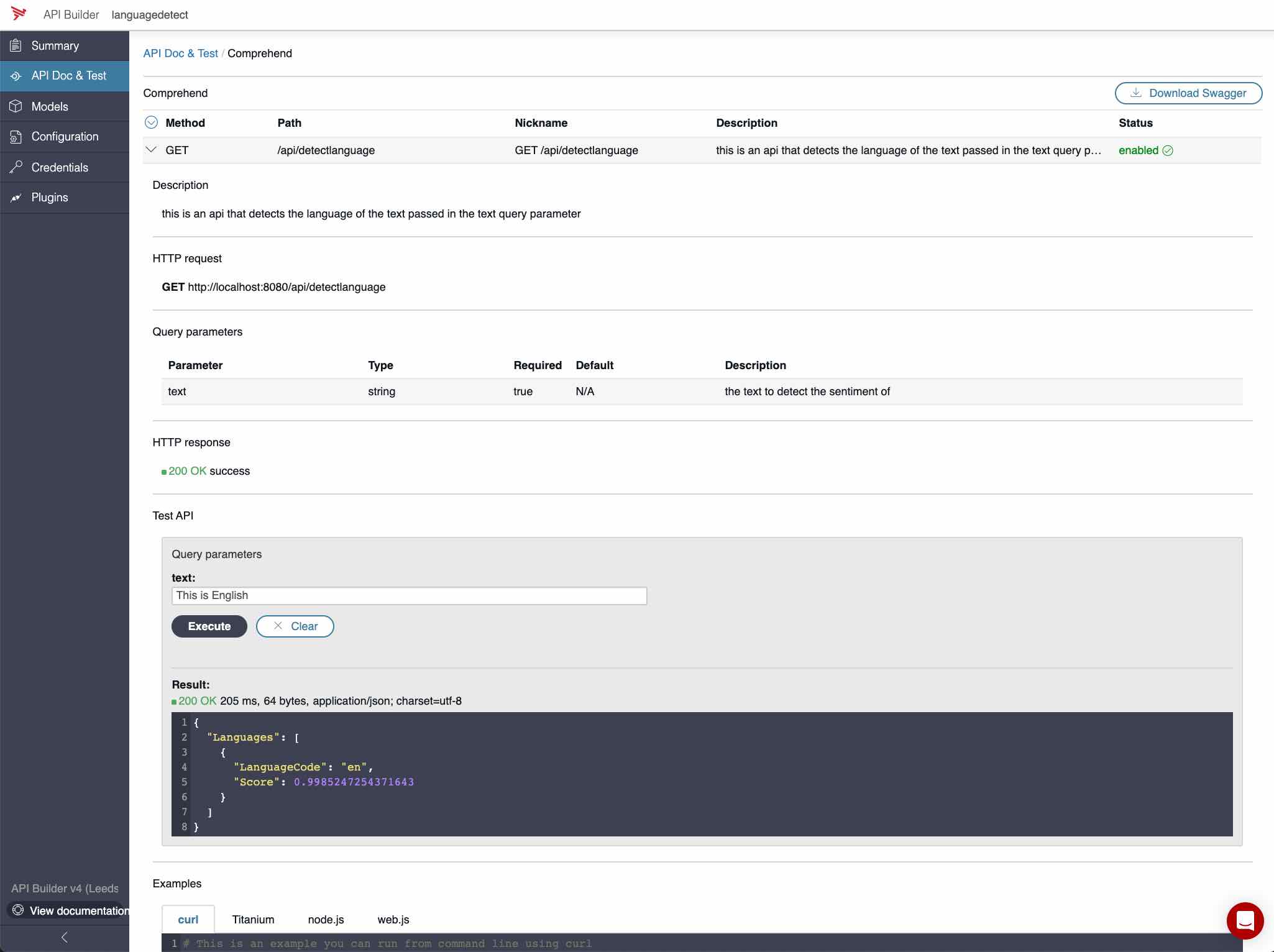
I passed in the following text:
“This is English”
The response shown below indicates that the input text was English (en):
{ "Languages": [ { "LanguageCode": "en", "Score": 0.9985247254371643 } ] }
If I pass in the following text:
“Esto no es ingles”
The response shown below indicates that the input text was Spanish (es):
{ "Languages": [ { "LanguageCode": "es", "Score": 0.913871169090271 } ] }
Publish Your API
You can follow the instructions here to create a Docker image and deploy in any docker platform or to publish to the Axway’s platform runtime service, ARS.
Summary
In this blog post, we described a method of creating APIs from a service that does not provide a REST API but does provide a Javascript SDK like the AWS Comprehend natural language processing (NLP) service. We used the API Builder’s custom API feature and exposed an API to detect the language of a string of text.
Follow us on social