Axway Integration Builder – Creating Reusable Code
When creating your Integration Builder Flows, you will find that you may need to create a method and use it in multiple steps in your flow. For example, you may have a method to extract a JSON object from an array of objects (e.g., the reply of a connector step) and you may need this in several steps. This post describes one means of accomplishing this.
We’ll use a JS Script step to create the methods early on in our flow and assign the methods to the config object so they are available in the other steps in your flow.
The idea is as follows:
* Create a JS Script step that defines all your methods that you want to access in other steps
* Add the methods to the config object
* Make this one of the first steps in your flow so that it will be available to all subsequent steps
* Access the methods in your other steps using the config.methodName syntax
Create a JS Step to Define your Methods
See the screen shot and code sample below that defines a JS Step, e.g. setMethods, for your methods and assigns them to the config object:
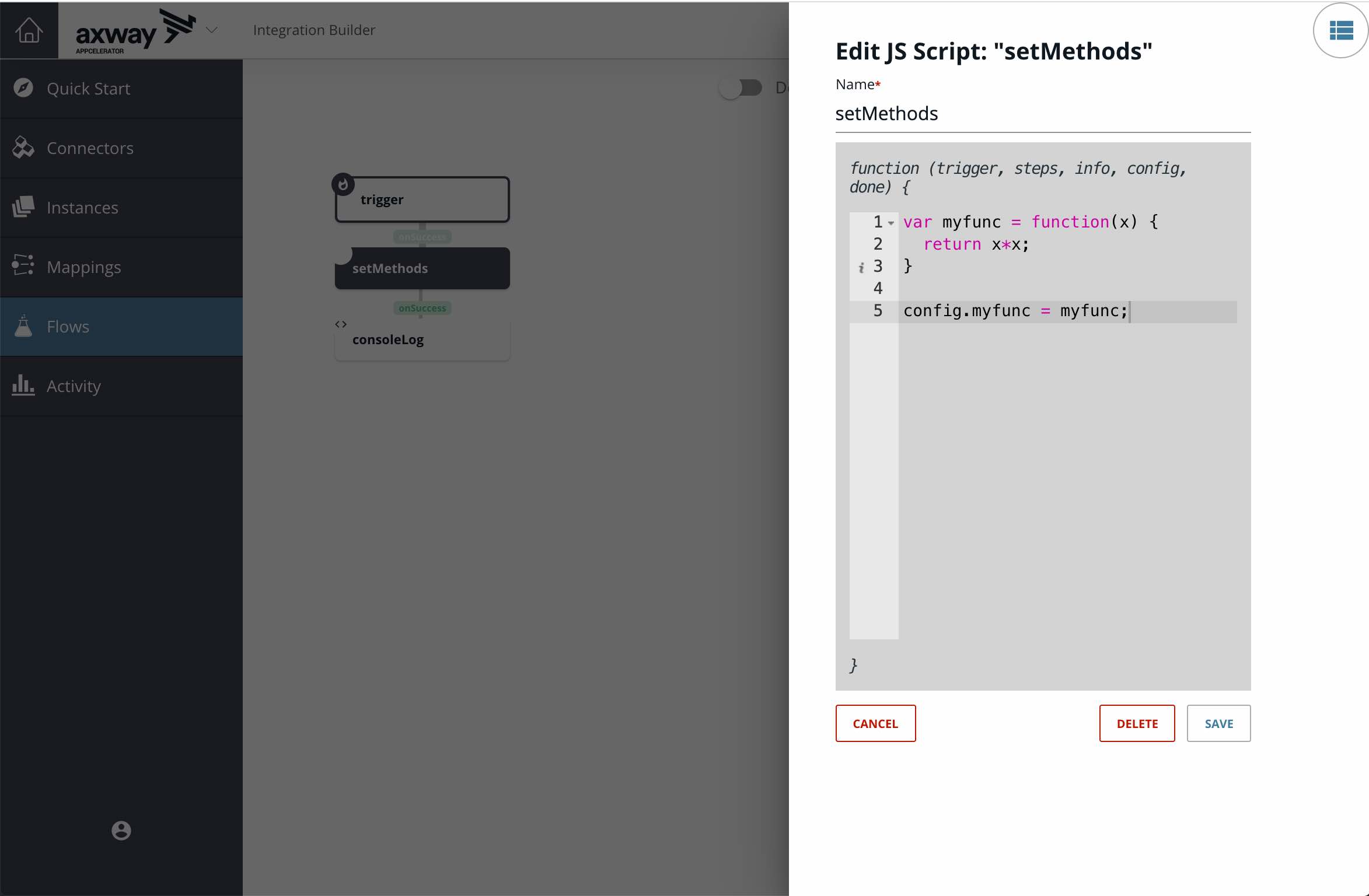
var myfunc = function(x) { return x*x; } config.myfunc = myfunc;
Note: You can assign multiple methods as well as variables to the config object. In the example above I am assigning just one method to config for clarity
Access the Methods
See the screenshot and code sample below that accesses the variables and methods in another step, for example:
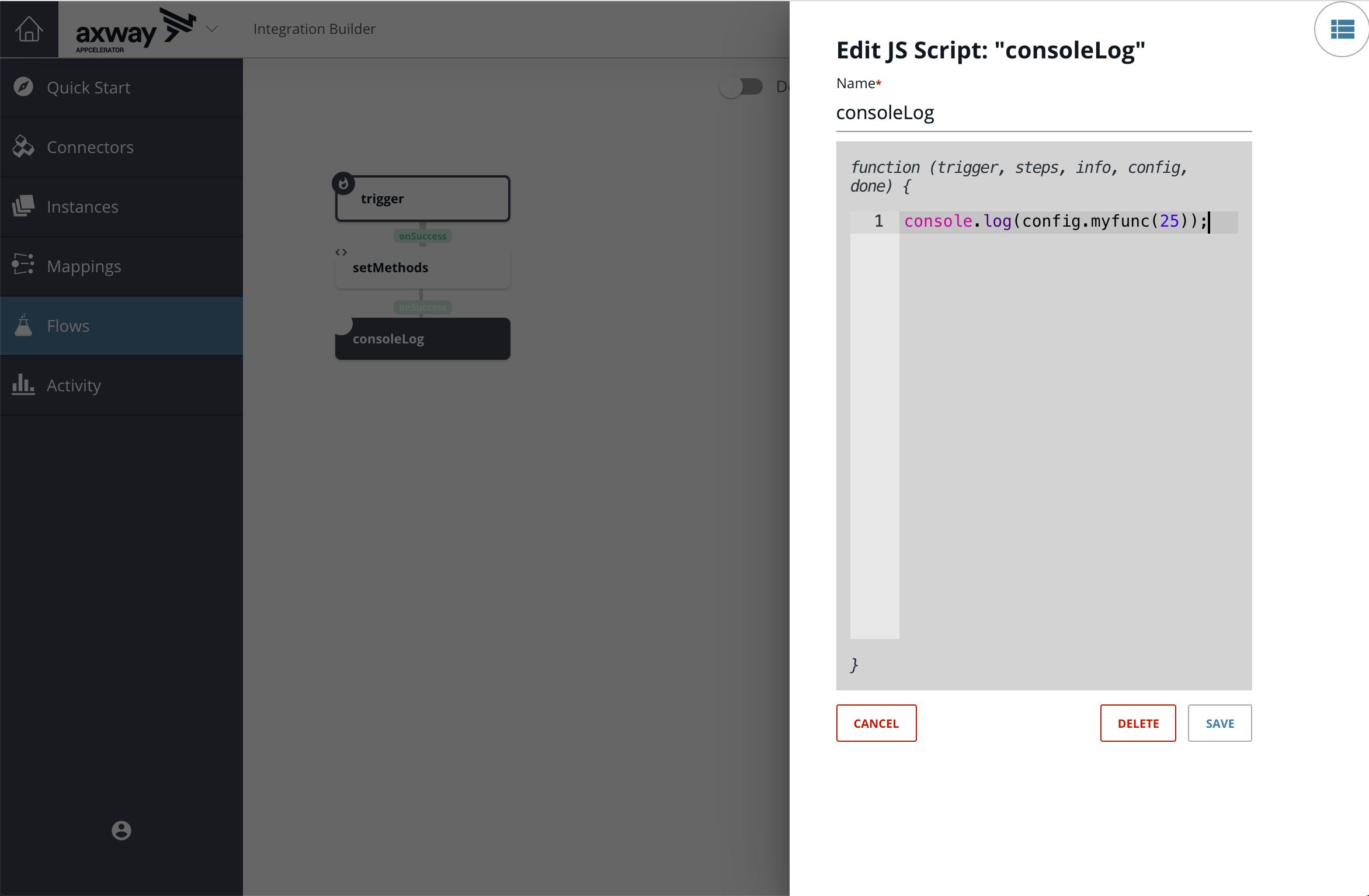
console.log(config.myfunc(25));
Check Executions
Trigger your flow and view the console.
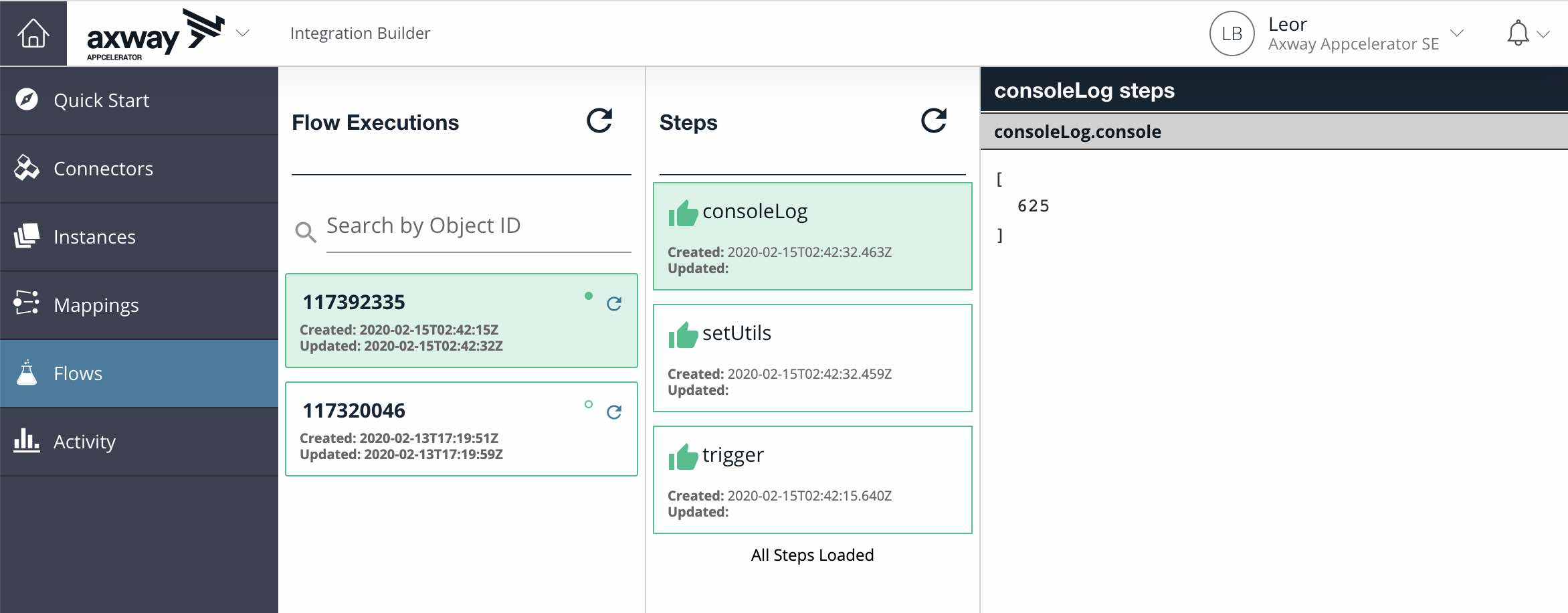
Optional
As an optional step, if you have several methods and global variables, you may want to encapsulate them in a separate object and assign that to the config object as follows:
var myfunc1 = function(x) { return x*x; } var myfunc2 = function(x) { return x*x*x; } var utils = { myfunc1 = myfunc1, myfunc2 = myfunc2 } config.utils = utils;
Then we can access the methods as follows:
console.log(config.utils.myfunc1(25)); console.log(config.utils.myfunc2(50));
Summary
We explored a technique to define and use methods across your flow without duplicating any code. This will help you create a cleaner code that is easier to maintain.
Learn how to create an Integration Builder Connector to an API Builder Microservice.
Follow us on social