API headers are extra information for each API call you make. They represent meta-data associated with an API request and response such as info about the request and response body, response caching and request authorization.
For example, Amazon Echo Alexa skills send two custom headers, SignatureCertChainUrl and Signature-256, with each webhook API call to your Alexa skill implementation that you must use to verify that the request was sent by Alexa.
In this post, we’ll look at how to read request headers as well as set response headers in an API Builder OpenAPI flow.
Request headers
In your API Builder OpenAPI flow, you can use the JSONPath $.request.headers[Header Name] to access a request header. For example, you can use $.request.headers[“x-customheader”] to access the request header x-customheader as shown below.
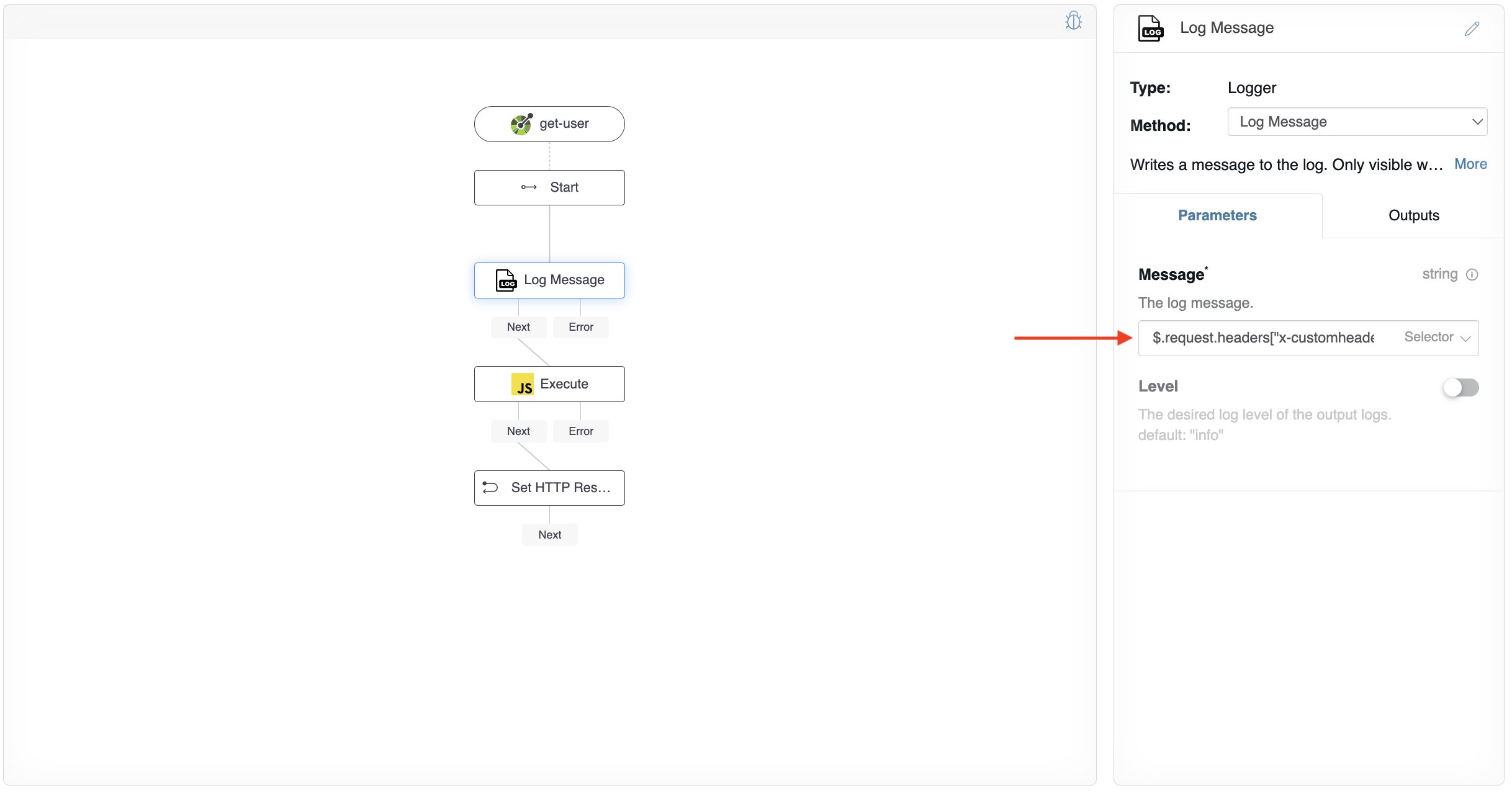
Response headers
The HTTP Response flow node has a Headers field where you can provide a JSON object of Key-value pairs of additional headers that you’d like sent with your response.
For example, the following JavaScript flow node sets the x-anothercustomheader header to a value. In this simple example, we are simply appending “,1234” to the x-customheader value.
async function code(data, logger) {
let headers = {
"x-anothercustomheader": data.request.headers["x-customheader"]+',1234'
};
return headers;
}
Then we can reference the JavaScript flow node output, $.result, in our HTTP Response flow node as shown below:
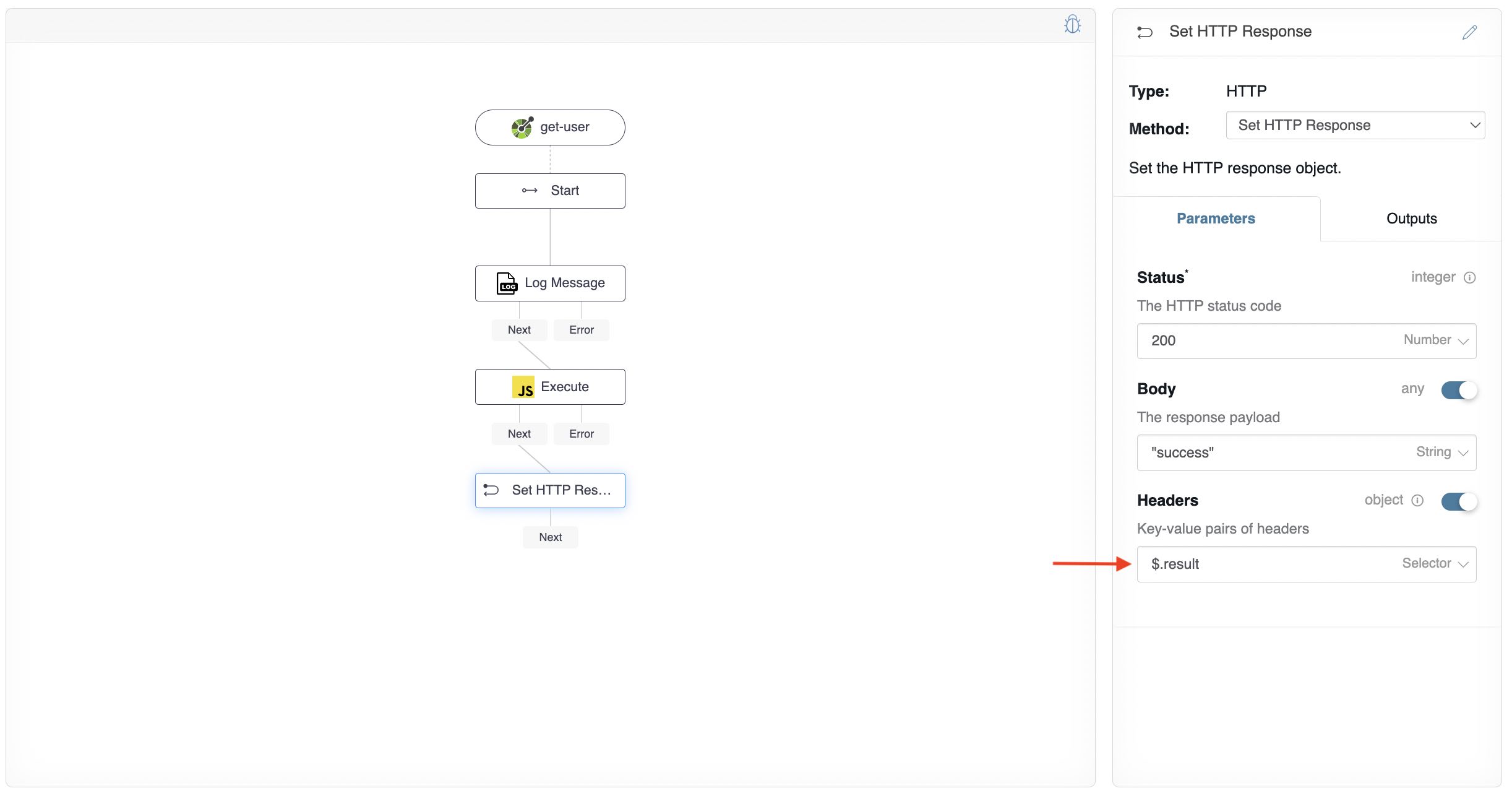
Note that I am setting the response body to “success” since this is just a mock API to illustrate accessing headers.
Putting it all together
You can see in my Stoplight API design below a request header, x-customheader, as well as a response header, x-anothercustomheader, for the GET /user
method:
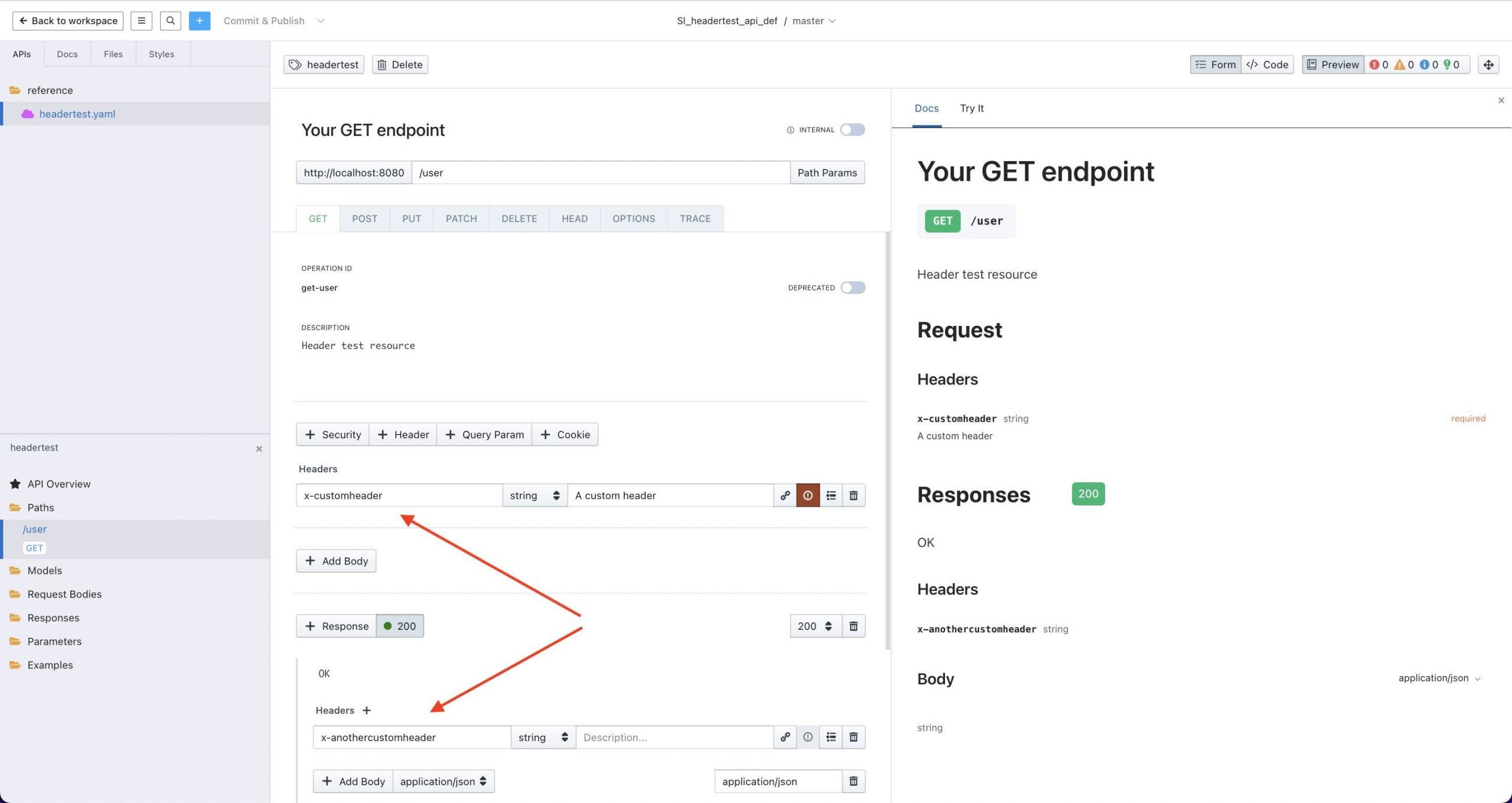
I imported the OpenAPI 3.0 spec into API Builder and created a simple mock API flow shown below:
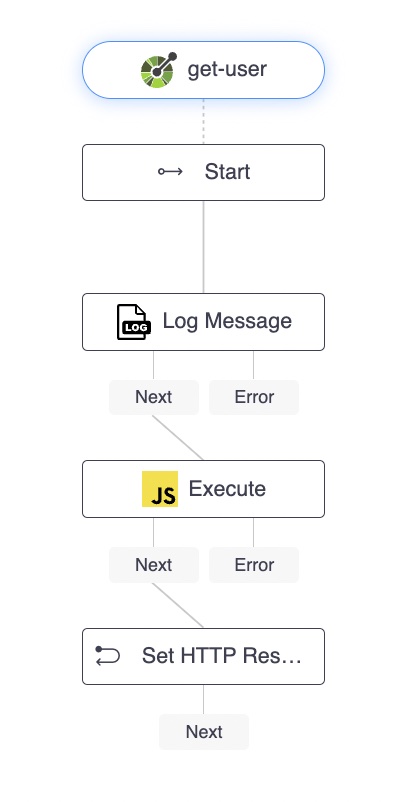
The API Builder project can be found here.
If I call the API using the following curl command which sets x-customheader to ‘aaa’:
curl -is -H "accept: application/json" -H "x-customheader: aaa" -X GET "http://localhost:8080/api/user"
I get the following response which has a response header, x-anothercustomheader, with a value of ‘aaa,1234′:
HTTP/1.1 200 OK
x-xss-protection: 1; mode=block
x-frame-options: DENY
surrogate-control: no-store
cache-control: no-store, no-cache, must-revalidate, proxy-revalidate
pragma: no-cache
expires: 0
x-content-type-options: nosniff
server: API Builder/4.89.0
request-id: c5bdf1d0-130b-42b7-bf75-965847a22b5b
start-time: 1652389384455
x-anothercustomheader: aaa,1234
content-type: application/json; charset=utf-8
response-time: 3
content-length: 9
Vary: Accept-Encoding
Date: Thu, 12 May 2022 21:03:04 GMT
Connection: keep-alive
Keep-Alive: timeout=5
"success"
Summary
In this post, we saw how to read request headers as well as how to set response headers in an API Builder OpenAPI flow.
Need help with API Builder? Extend your skills with Axway University.
Follow us on social